Python functions bring sanity to coding chaos. To create one, start with the keyword 'def' followed by a function name, parentheses for any parameters, and a colon. Then indent the code block below it. Add a docstring to explain what your function does—future you will thank present you. Functions can accept different types of arguments and return values when called. The magic happens when you start combining these powerful little blocks.
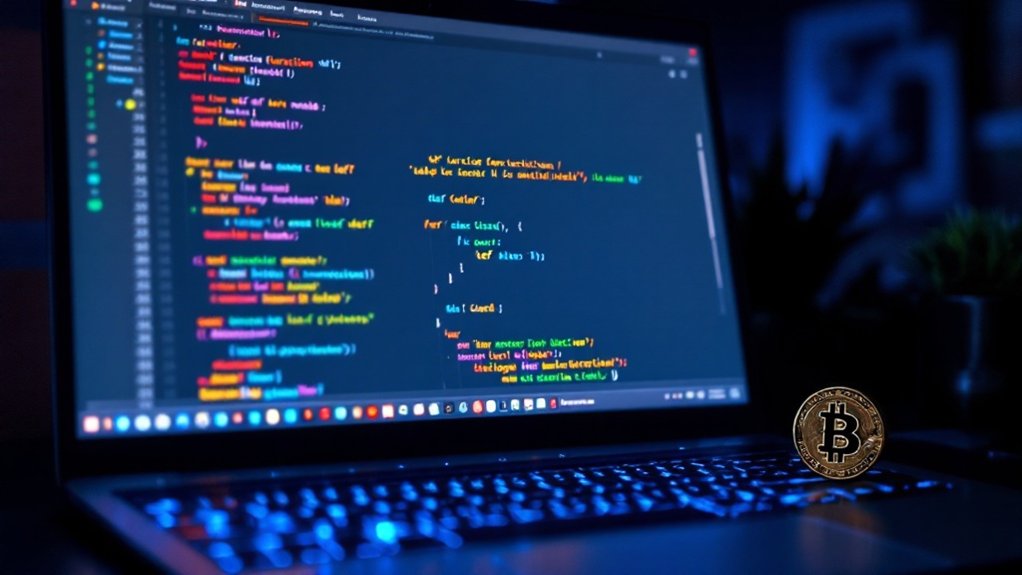
Functions power the Python ecosystem. They're the building blocks that make code modular, reusable, and maintainable. Every Python programmer needs to master them. No exceptions.
Creating a function starts with the 'def' keyword, followed by a name that actually makes sense. Seriously, don't name your function 'do_stuff' – nobody knows what that means. After the name comes parentheses for parameters, then a colon. The colon matters. Forget it, and Python throws a fit.
Indentation isn't optional in Python. It defines the function body. Four spaces is standard. The function body can start with a docstring – a description of what the function does. It's technically optional, but skipping it is lazy. The body contains the actual code that executes when the function is called. Functions can print output directly when executed without requiring a return value. At the end, a return statement sends values back to wherever the function was called from.
Parameters make functions flexible. They come in several flavors. Positional arguments must be passed in order. Keyword arguments use names for clarity. Default values handle cases when arguments aren't provided. Some functions need variable numbers of parameters – that's where arbitrary arguments shine. Many modern APIs use RESTful endpoints to handle different parameter types efficiently. Just like data preparation in machine learning, proper parameter handling ensures your function works with clean, properly formatted inputs.
Calling a function is straightforward. Type the name, add parentheses, include any needed arguments, and you're done. The function executes, and any return value can be stored in a variable. You can also run your function by executing it with the python filename.py command. Easy.
Python offers advanced function features for the ambitious. Lambda functions create small, anonymous functions in a single line. Generators yield values one at a time. Decorators modify function behavior. Closures remember values even after a function finishes. Recursion lets functions call themselves – mind-bending but powerful.
Good functions follow patterns. They're clearly named. They're documented with docstrings. They use type hints to prevent bugs. They're thoroughly tested. They're periodically refactored. They do one thing well. Get these basics right, and your Python code will actually work. Imagine that.
Frequently Asked Questions
How Do I Handle Errors and Exceptions in Python Functions?
Developers handle errors in Python with try-except blocks. Simple concept, essential execution. Code goes in the try block, error handling in except.
Want specificity? Catch particular exceptions like ValueError—not just blanket except statements. That's amateur hour.
For cleanup operations, finally blocks execute regardless of errors. Advanced users implement custom exceptions and logging. Some throw in context managers or decorators for automation.
Error handling isn't fancy, but it's non-negotiable for robust code. Programs break. Deal with it.
Can I Use Default Parameters in Python Functions?
Yes, Python functions fully support default parameters. They're defined using the syntax parameter=value in function declarations.
Default parameters must come after regular parameters. Super useful for making arguments optional. Just watch out for mutable defaults—they can cause weird bugs.
Lists as defaults? Bad idea. They persist between function calls.
Default parameters make code cleaner and more flexible. No need to pass every argument when you don't want to. Pretty handy feature, actually.
What's the Difference Between Arguments and Parameters?
Parameters are placeholders in function definitions. Arguments are the actual values you pass when calling the function. Simple as that.
Parameters set up what the function expects – like a blueprint waiting to be filled in. Arguments are what you're actually throwing at the function during execution. One's theoretical, one's practical.
Parameters appear in the function declaration, arguments show up at runtime. They're related but distinct. Parameters receive; arguments are received.
How Do I Document My Functions With Docstrings?
Docstrings are Python's built-in documentation system. To use them, add triple-quoted strings right after function definitions. They explain what the function does, its parameters, and return values.
'''python
def add(x, y):
"""Adds two numbers and returns the result.
Args:
x: First number
y: Second number
Returns:
Sum of x and y
"""
return x + y
'''
Access them with 'help(add)' or 'add.__doc__'. Simple, yet effective.
Most devs use Google or NumPy style. Documentation matters.
When Should I Use Lambda Functions Instead of Regular Functions?
Lambda functions shine for quick, one-line operations.
They're perfect when you need a throwaway function for filtering data or simple transformations. Regular functions? Better for complex logic.
Lambda's limitations are real – single expressions only, no statements, harder debugging. Great with higher-order functions like map() and filter(). They keep code concise when the operation is simple.
But remember: they're not a replacement for regular functions. Just a different tool for specific situations.