Python virtual environments solve dependency nightmares by creating isolated spaces for projects. To set one up, run "python3 -m venv /path/to/newenv" and activate it with "source /path/to/env/bin/activate" on Linux/Mac or "patho\env\Scripts\activate" on Windows. Your prompt changes, showing it worked. Install packages with pip without affecting your system Python. When done, type "deactivate" and you're out. The rest of the process gets even better.
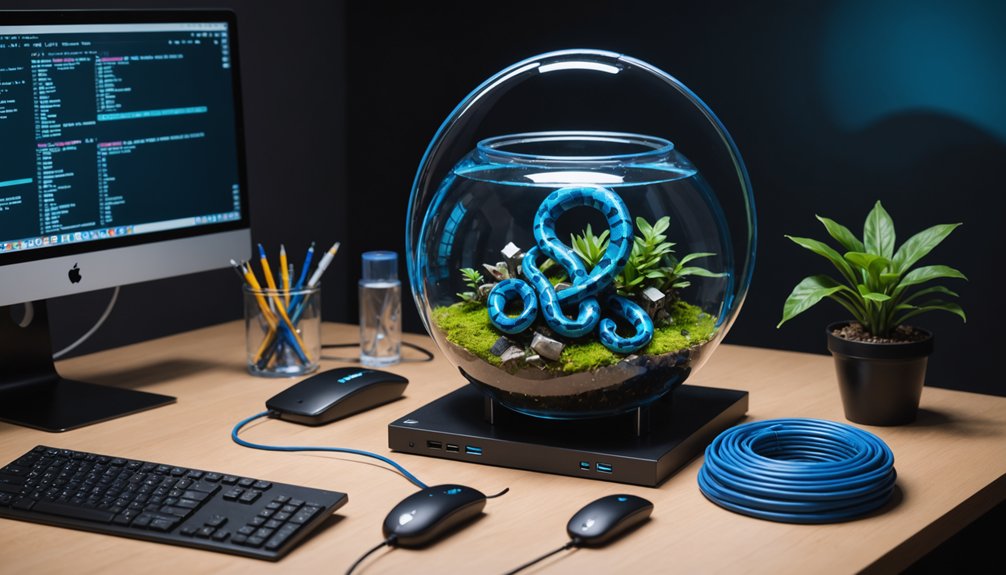
Every Python developer faces dependency nightmares eventually. Projects clash. Libraries fight. Version conflicts turn clean code into a tangled mess. It happens to the best coders. Python's solution? Virtual environments. They're isolated spaces where Python code can run without messing with other projects or the system Python installation. Brilliant stuff, really.
Virtual environments solve real problems. They keep projects separated, with their own dependencies and versions. No more "it works on my machine" excuses. They make projects portable and reproducible across different systems. Testing becomes easier too. Want to try a new library version without breaking everything? Virtual environment to the rescue. Similar to how function docstrings help maintain clean, reusable code, virtual environments ensure project organization and maintainability.
Python's virtual environments aren't just nice to have—they're essential armor against the chaos of dependency conflicts.
Creating one is dead simple. Just run "python3 -m venv /path/to/newenv" and the system does the heavy lifting. Each environment includes a pyvenv.cfg file that references the base Python installation. The command generates directories for binaries, libraries, and other necessities. Windows users get a "Scripts" directory instead of "bin" because, well, Windows has to be different. Many developers use virtual environments to create isolated spaces for ChatterBot training and other AI applications.
The newer "venv" tool is preferred over the older "virtualenv" for Python 3.3+. Backward compatibility matters, though, so "virtualenv" still exists for dinosaur Python versions.
Activation comes next. On Linux or macOS, type "source /path/to/env/bin/activate". Windows users need "pathoenvScriptsactivate". The terminal prompt changes, showing the environment name. That's your clue that it's working. Check with "which python" to confirm.
Once activated, package management becomes a breeze. Install packages with pip. Track dependencies in a requirements.txt file. Update, remove, or freeze package versions as needed. The system Python stays clean. Other projects remain untouched. Magic.
When finished, just type "deactivate". The environment name vanishes from the prompt. Back to regular Python. You can also list currently installed packages with pip list to see what's in your environment. Done with the environment completely? Delete the directory. That's it. No complicated uninstall procedures. No registry entries to purge. Just delete and move on. Clean, simple, effective.
Frequently Asked Questions
How Do I Manage Different Versions of the Same Package?
Managing different versions of the same package is a real headache. Virtual environments save the day. Each project gets its own sandbox—no more package wars.
Developers use tools like pip, uv, or conda to install specific versions in isolated environments. Requirements.txt files track what's needed.
It's simple math: one project, one environment, zero conflicts. No more dependency hell. Problem solved.
Can I Use Virtual Environments With Jupyter Notebooks?
Yes, Jupyter Notebooks work great with virtual environments. Users can create separate environments, install ipykernel, and register them as Jupyter kernels.
Boom—isolated dependencies for each project. No more package conflicts. Simply activate the environment, run 'python -m ipykernel install –user –name=myenv', and select your custom kernel in Jupyter.
Different projects, different packages, same Jupyter installation. Clean, organized, efficient.
How Do I Share My Virtual Environment With Team Members?
Sharing virtual environments is simple.
Don't copy the environment folder—that's amateur hour. Instead, generate a requirements.txt file using 'pip freeze > requirements.txt' and distribute that.
Team members create their own virtual environments, then run 'pip install -r requirements.txt'. Done.
For extra credit, create a batch script to automate the process. Platform differences can be tricky, so document any special cases.
What's the Difference Between Venv, Virtualenv, and Conda?
Venv is Python's built-in environment tool. Simple, lightweight, comes with Python.
Virtualenv? Same deal, but third-party. Old-school choice before venv became standard.
Conda plays in a different league. Cross-platform powerhouse that handles multiple languages, not just Python. Popular with data scientists.
Manages complex dependencies that would make venv cry.
Bottom line: Python-only project? Venv works fine. Dealing with scientific packages or multiple languages? Conda's your friend.
Can I Use Virtual Environments in Production Deployments?
Virtual environments aren't just for development—they're production powerhouses too. Companies deploy them daily. They isolate dependencies, preventing conflicts that could crash systems.
Version control? Check. Reproducibility across servers? Absolutely. Security benefits are massive; no more exposing system-wide packages to vulnerabilities.
Deployment automation becomes straightforward with consistent environments. Plus, rollback mechanisms are easier to implement. Production-ready? You bet they are.