Python coding starts with clean syntax and minimal complexity. No semicolons needed here. Indentation isn't just for show—it defines code blocks. Variables work without declarations, while control structures use straightforward keywords like "if," "elif," and "else." Comments use the hashtag symbol. Python handles data structures effortlessly through lists, dictionaries, and sets. Functions begin with "def" and offer reusable code chunks. The extensive community makes problem-solving a breeze. Dive deeper and watch those curly braces disappear.
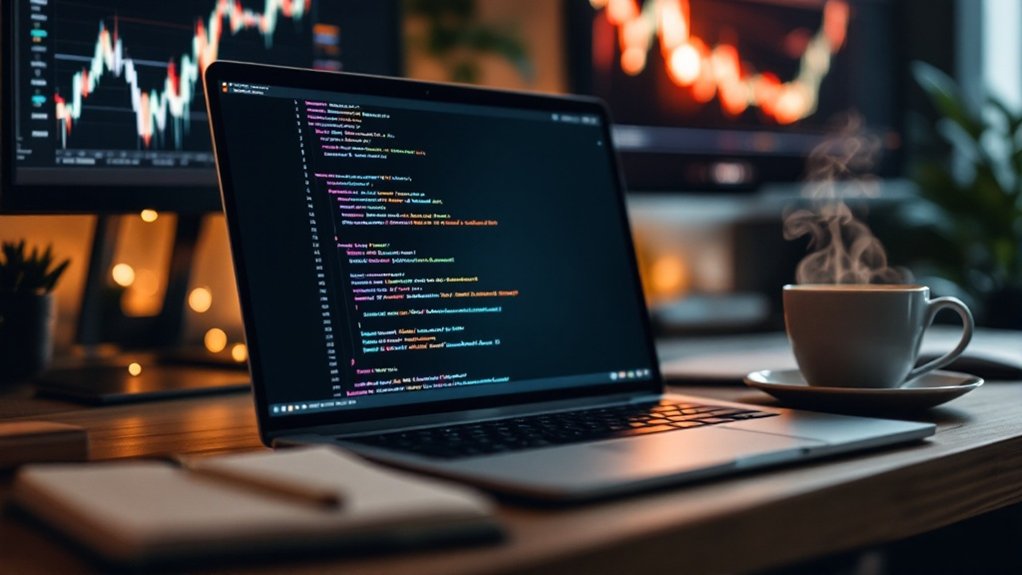
In a world dominated by digital innovation, Python stands tall as one of the most popular programming languages today. It's not hard to see why. The language is ridiculously readable and easy to use. No wonder it's infiltrated web development, data science, machine learning, and automation. Beginners have it good – tutorials and courses are everywhere. W3Schools. LearnPython.org. Take your pick.
Setting up is a breeze. Visual Studio Code works. So do Jupyter Notebooks. Choose whatever feels right. The Python community? Massive. Active. Always ready to help with extensive libraries and support forums. Good luck finding a coding problem someone hasn't already solved.
Setting up Python? Painless. Pick your editor, tap into the community. Someone's already solved your next problem.
Let's talk basics. Indentation in Python isn't just for show – it's how the language defines code blocks. Forget it, and your code breaks. Simple as that. Comments use the '#' symbol. They're for explaining your code to other humans. Or your future self, who'll stare blankly at that "perfectly clear" function you wrote at 3 AM.
Variables? Just assign them. 'a = 5'. Done. Python handles integers, floats, strings, and booleans without breaking a sweat. Need to convert between types? Functions like 'int()', 'float()', and 'str()' have got your back. Variable names must follow specific rules – they can contain letters, numbers, and underscores but cannot start with numbers or use reserved keywords like 'if' or 'for'. Python treats variables as object labels that point to data rather than containers that hold values.
Control structures keep things interesting. 'If', 'elif', and 'else' statements make decisions. Loops repeat code until you tell them to stop. Functions package code for reuse – define them with 'def', call them when needed, and return values if you want. Many developers use Python to build RESTful APIs with frameworks like Flask and FastAPI. Python's Beautiful Soup library makes web scraping a breeze for extracting data from HTML pages.
Input comes from users with the 'input()' function. Output goes to the console with 'print()'. Reading and writing files? Python handles that too.
For the ambitious, lists store collections of items. Dictionaries keep key-value pairs. Sets maintain unique items.
Python's not perfect. But it's close. Get the syntax right, and you're already ahead of most beginners. The rest? Practice. Experiment. Break things. Fix them. That's coding 101 for you.
Frequently Asked Questions
How Does Python Compare to Javascript for Beginners?
Python beats JavaScript for beginners. Period. The syntax is cleaner, with fewer structural rules to trip you up. No semicolons, no messy variable declarations. Python reads almost like English—seriously.
JavaScript's complexity and asynchronous nature? Good luck debugging that nightmare as a newbie. Both have great online resources, but Python's straightforward approach makes the learning curve less steep.
Plus, Python opens doors to data science while JavaScript primarily serves web development. Choose your poison.
Can I Build Mobile Apps With Python?
Yes, Python can build mobile apps, though it's not the most obvious choice.
Frameworks like Kivy, BeeWare, and PyQt make it possible. Cross-platform development is a major perk – write once, deploy everywhere. Nice.
Performance isn't stellar compared to native solutions, and deployment can get messy.
Python excels for data-heavy applications and rapid prototyping. Not ideal for graphics-intensive games or apps needing deep hardware integration.
Works, but with trade-offs.
Is Python Suitable for Game Development?
Python for game development? Yes, with caveats.
It's great for beginners and indie devs. Perfect for 2D games and prototyping. Pygame, Panda3D, and Ursina make life easier.
But let's be real—it's not winning any speed contests. AAA titles? Forget about it. Performance issues are a thing.
Python shines with AI integration though. Many developers use it for server-side logic in bigger games.
Not the fastest horse in the race, but definitely rideable.
How Much Math Knowledge Do I Need for Python?
For basic Python, middle school math is enough. Addition, subtraction, multiplication, division – the usual suspects. Variables and simple equations help too.
Want to dive deeper? Different fields need different math skills. Data science? Linear algebra's your friend. Graphics? Hello, geometry. Machine learning? Statistics and calculus enter the chat.
The average Python programmer isn't solving complex equations daily. But understanding basic math concepts makes problem-solving way easier. Math and programming are cousins, after all.
Should I Learn Python 2 or Python 3?
Python 3 is the only logical choice in 2023. Period.
Python 2 has been dead since January 2020 – officially buried with no more security updates. Industry has moved on.
Modern libraries? They're all Python 3 now. Learning an obsolete language version would be like studying VHS repair in the Netflix era.
Python 3 offers cleaner syntax, better memory management, and actual future prospects. Plus, it handles Unicode properly.
The job market doesn't want Python 2 dinosaurs.