One-hot encoding transforms categorical data into a binary format machines can actually understand. In Pandas, the get_dummies) function handles this conversion effortlessly. Each category gets its own column with 1s marking presence and 0s for absence. It prevents algorithms from assuming relationships between unrelated categories. The technique creates clean numerical data but watch out—it can explode your feature space with too many columns. Proper preprocessing makes all the difference between mediocre and stellar model performance.
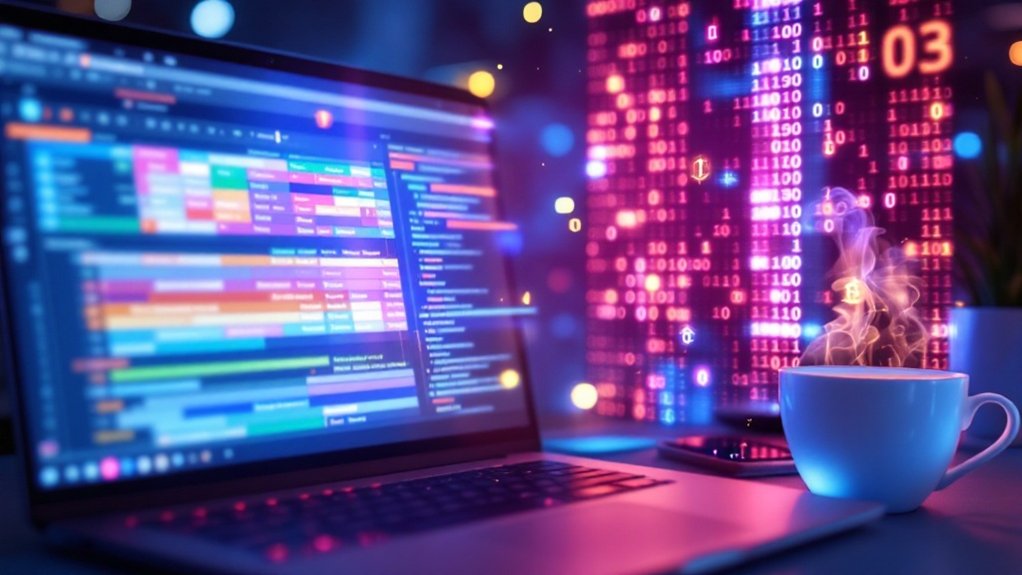
When working with machine learning models, categorical data presents a unique challenge. Machines don't understand text. They crave numbers. That's where one-hot encoding steps in—transforming those pesky categorical variables into something useful for algorithms to digest.
One-hot encoding converts categorical data into binary format. It's that simple. No more struggling with text variables that make your models throw tantrums. Each category becomes its own column. Present? Value is 1. Not present? Zero. Done.
Pandas makes this process ridiculously straightforward with its 'get_dummies()' function. No need for complex code or mental gymnastics. Just pass your dataframe, and watch the magic happen. For example, 'pd.get_dummies(df, dtype=int)' does all the heavy lifting, creating those binary columns automatically.
The beauty of one-hot encoding? No implied ordinal relationships. Categories like "red," "blue," and "green" don't suggest any ranking—unlike numerical encoding, which might accidentally imply that "green" (encoded as 3) is somehow better than "red" (encoded as 1). Machine learning algorithms can be embarrassingly literal sometimes. Before diving into encoding, it's essential to begin with problem definition to ensure you're using the right approach for your specific machine learning task.
Sure, there are challenges. One-hot encoding creates a new column for EVERY category value. Dataset with 50 countries? Congrats, you've just added 50 new columns. The curse of dimensionality is real, folks. As experienced data science experts frequently discuss in related articles, this explosion of features can impact model performance. It's important to remember that after encoding, you should drop original columns to prevent multicollinearity issues in your models.
Scikit-learn offers its own implementation through 'OneHotEncoder' with more bells and whistles. It handles unknown values better and integrates seamlessly into machine learning pipelines. Fancy. Z-score standardization can be applied after encoding to ensure all features contribute equally to model training.
Data scientists everywhere rely on this technique daily. It's part of the standard preprocessing toolkit—like brushing your teeth before a date. Essential, not optional.
When implementing one-hot encoding, follow a simple process: identify categorical columns, apply encoding, check dimensionality, handle unknowns, and proceed with your machine learning workflow. Nothing to it.
Alternatives exist. Label encoding. Binary encoding. But for categorical variables without inherent order? One-hot encoding remains king. No contest.
Frequently Asked Questions
How Does One-Hot Encoding Handle Missing Values?
One-hot encoding doesn't handle missing values by itself. Period. It's a transformation technique, not a cleaning one.
Before encoding, data scientists need to address those pesky missing values through imputation (using mean, median, or mode values), deletion of rows, or creating a separate category for them.
Scikit-learn's OneHotEncoder has a 'handle_missing' parameter that can help, but the preprocessing step is essential.
No cleaning, no reliable encoding. Simple as that.
What's the Difference Between One-Hot Encoding and Dummy Coding?
The main difference is simple. One-hot encoding creates binary columns for all categories. Dummy coding drops one category to avoid multicollinearity.
Both transform categorical data into numbers, but dummy coding is more efficient. One-hot uses 'pd.get_dummies()' while dummy uses 'pd.get_dummies(drop_first=True)'.
Statisticians love dummy coding for regression models. Machine learning folks? They often don't care either way. The model results are typically identical anyway.
When Should I Use Label Encoding Instead of One-Hot Encoding?
Label encoding works best for ordinal data – categories with a natural ranking. Think: Small, Medium, Large. Makes sense, right?
Decision trees and random forests handle it well. One-hot encoding is better for nominal data where categories have no inherent order. Using label encoding on nominal data? Bad idea. It creates false relationships.
Models like neural networks and logistic regression get confused. Dataset size matters too. One-hot encoding bloats your features. Sometimes that's a deal-breaker.
How Can I Reverse One-Hot Encoding Back to Categorical Data?
To reverse one-hot encoding, you've got options.
With scikit-learn, it's straightforward—just use the 'inverse_transform()' method on your OneHotEncoder object.
Pandas users got lucky in version 1.5.0 with the new 'from_dummies()' function. Before that? Tough luck. You'd need a manual approach.
Both methods require the original column names. Category handling matters here. Get it wrong, and your data's toast.
Testing on small datasets first? Smart move.
Does One-Hot Encoding Increase Model Training Time Significantly?
One-hot encoding definitely increases model training time. It's basic math. More columns means more calculations. Period.
The impact varies though – small datasets? Not a big deal. Massive ones with tons of categories? You're in for a wait.
Some algorithms handle it better than others. Decision trees? They're fine. Linear models? Not so much.
Smart techniques exist to mitigate the slowdown – dimensionality reduction, sparse matrices, feature selection.
Worth it for the accuracy boost? Usually. But there's always a tradeoff.