Renaming columns in Pandas is dead simple. Use df.rename) with a dictionary mapping old names to new ones: df.rename(columns={”old”: “new”}). For wholesale changes, try df.columns = [“name1”, “name2”] or set_axis(). The inplace=True parameter updates the original DataFrame—skip it if you need the old version. Case sensitivity matters, so watch your caps. Pandas makes column renaming straightforward, but the details make all the difference.
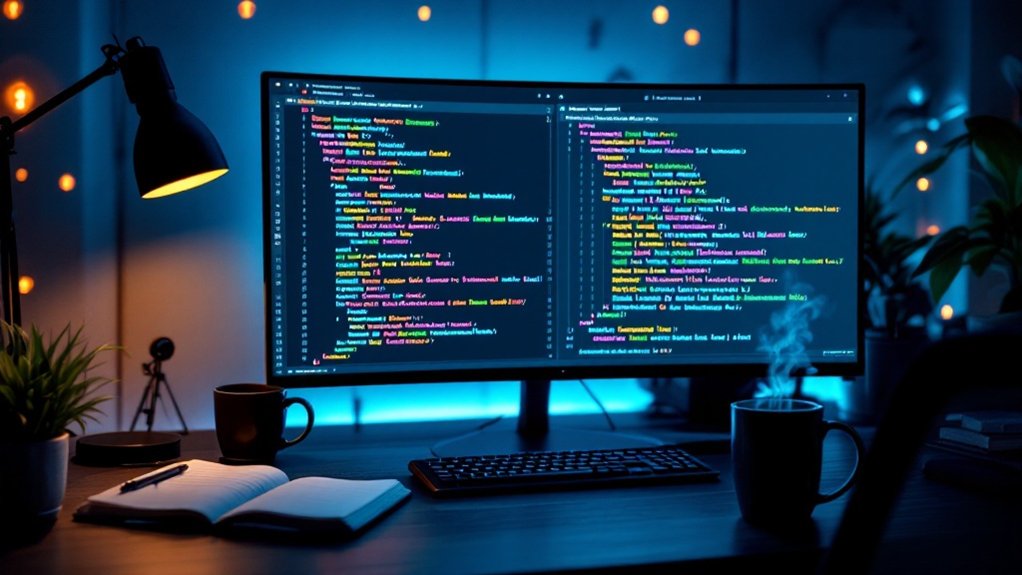
Wrangling messy data often means fixing terrible column names. Let's face it: data rarely arrives in pristine condition. Those auto-generated headers from spreadsheets? Useless. Cryptic variable names from ancient databases? Nightmare fuel. Thankfully, the Pandas library in Python offers several ways to transform these monstrosities into something actually readable.
First things first, you need Pandas installed. A quick "pip install pandas" followed by "import pandas as pd" gets you started. No Pandas, no party. That's just how it works. Just like data preprocessing is essential for AI model building, properly named columns form the foundation of effective data analysis.
Before diving into data cleaning, ensure Pandas is ready to roll. No installation, no transformation magic.
The most common approach uses the "rename()" method with a dictionary. Old names go as keys, new names as values. Simple. Want to rename a single column? Easy. Multiple columns? Just add more key-value pairs to your dictionary. The beauty here is you only touch what needs fixing—everything else stays put. Similar to Python's dictionary get method, this approach helps avoid errors when working with column names.
For the scorched-earth approach, replace all column names at once by assigning a new list to "df.columns" or using "set_axis()". But watch out! The list length better match your column count exactly. One name short and your code breaks. No warnings, just failure.
Sometimes you need more creative solutions. Functions can transform names systematically—capitalize everything, remove spaces, whatever. Lambda functions handle complex patterns. It's amazing how much cleaner data looks with consistent naming.
Need to target specific columns? Rename the last one with “df.columns[-1]” or pick any column by its index. You can even modify column names while importing your CSV files using the names argument. Conditional renaming works too, for those special cases where only certain columns need attention. Setting the inplace parameter to True ensures your DataFrame is modified directly without having to reassign it.
Remember that renaming is case-sensitive. One wrong capital letter and you'll spend an hour debugging. Been there, done that. Not fun.
The right column names make analysis infinitely easier. They're not just labels—they're the foundation of readable, maintainable code. Might seem tedious, but five minutes fixing names now saves hours of confusion later.
Frequently Asked Questions
How to Rename Columns Conditionally Based on Values?
To rename columns conditionally based on values, one can use several approaches in pandas.
Dictionary mapping works for straightforward cases. Lambda functions offer more flexibility—perfect for pattern matching or string manipulation.
For complex scenarios, custom functions with conditional logic are the way to go. Developers can iterate through the DataFrame, checking values and renaming accordingly.
Simple stuff for small datasets, potentially resource-intensive for larger ones. Testing is essential. No one wants column names that make zero sense.
Can I Rename Columns During Data Import?
Pandas doesn't support renaming columns directly during import. That's just how it is. Users must first import the data, then rename columns afterward using methods like 'rename()' or 'set_axis()'.
For example:
'''python
Import first
df = pd.read_csv('data.csv')
Then rename
df.rename(columns={'old_name': 'new_name'}, inplace=True)
'''
Some people find this annoying, but it actually gives more control.
Plus, it's more memory-efficient for large datasets.
How to Rename Columns With Special Characters?
To rename columns with special characters in pandas, use the 'rename()' method with a dictionary. Just map old names to new ones:
'''python
df.rename(columns={"column.with.dots": "new_name",
"column/with/slashes": "better_name"})
'''
Special characters can be tricky. They're perfectly legal in pandas, but they'll cause headaches later.
Especially with the 'query()' function. Escape them with backticks if needed:
'''python
df['column.with.dots']
'''
Simple as that.
Best Practices for Automating Column Renaming?
Automating column renaming? Simple stuff. Consistency is king. Pick a naming convention and stick with it. Functions are your friend. Create reusable scripts that standardize column names across datasets. No manual typing required.
For complex renaming, regex patterns work wonders. Documentation matters too. Write it down, or future-you will curse past-you. Regular testing prevents disasters.
And please, version control your code. Saves headaches when things inevitably break.
Does Renaming Columns Affect Performance With Large Datasets?
Renaming columns in pandas? Not a performance killer, even with big datasets. The operation is lightweight – just changing metadata, not touching the actual data. Quick and efficient.
No significant memory impact either. Methods like 'rename()' with a dictionary or direct column attribute modification work equally well.
Still, for absolutely massive datasets, it's smart to test first. But generally? No sweat. Column renaming won't be your bottleneck.