To import Excel files into R, first install R and RStudio. Install the 'readxl' package with 'install.packages("readxl")' and load it using 'library(readxl)'. Set your working directory with 'setwd()'. Import your file with 'read_excel("filename.xlsx")'. Specify sheets by name or number if needed. Check your data with 'head()' or 'View()'. File path errors are common troublemakers. Alternative packages exist if 'readxl' gives you grief. The full process reveals more nuances.
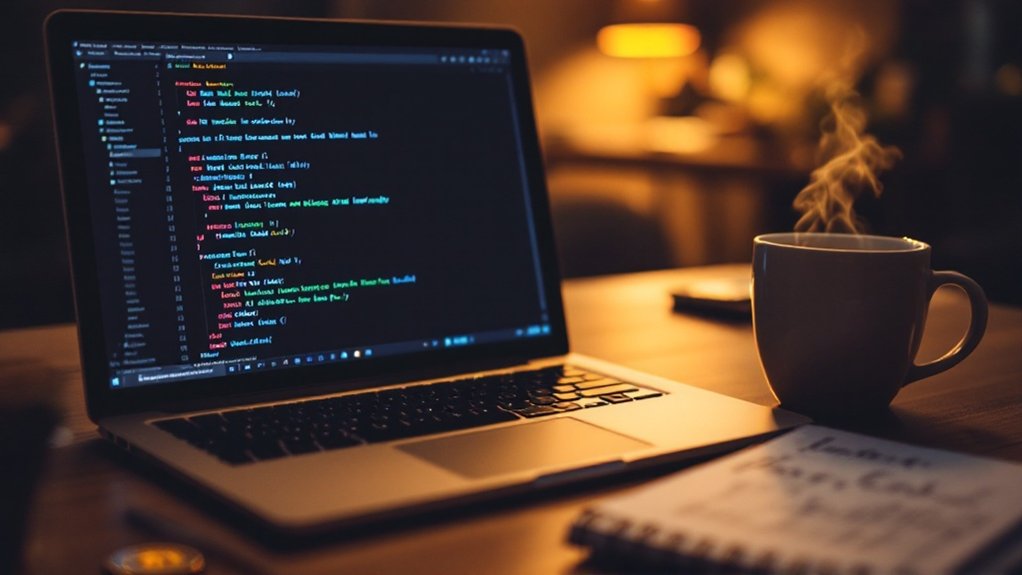
Struggling with Excel files and R? Let's be honest – it's not exactly rocket science. But it can be a pain. Especially when you're staring at that Excel spreadsheet, wondering how to get that data into R without losing your mind.
First things first. You need R. Download it from the official website. Then grab RStudio. These are non-negotiable prerequisites. Don't even think about proceeding without them.
Skip the fluff. Get R and RStudio installed first. These are your mandatory starting points.
The real MVP here is the 'readxl' package. It's designed specifically for importing Excel files – both .xls and .xlsx formats. Install it using the command 'install.packages("readxl")'. Then load it with 'library(readxl)'. Simple stuff, really.
For the GUI enthusiasts (you know who you are), RStudio makes life easy. Click 'Import Dataset' in the Environment pane, select 'From Excel…', browse for your file, and voilà! Options galore for tweaking your import settings. Preview the data before committing. Smart. Some users prefer to first convert to CSV format for easier handling.
Code warriors have a different path. After loading the package, set your working directory with 'setwd()'. Critical step. Don't skip it. Then use 'read_excel()' to import your file. If you're getting errors, check your current working directory with getwd() function. Remember to verify the data using functions like 'head()' or 'View()' to ensure everything imported correctly. Just like data preprocessing is crucial in AI model building, proper data verification is essential here. Check your data with 'head()' or 'View()'. Done.
Got multiple sheets? No problem. Specify which one using the 'sheet' argument. Use names or numbers. First sheet imports by default if you're feeling lazy.
Missing values? Specify NA identifiers during import. Clean afterward for consistency. Verify everything. Trust nothing.
Not a fan of 'readxl'? Alternatives exist. Try 'xlsx' or 'openxlsx'. They offer different features. Compare them. Choose wisely.
Watch out for common pitfalls. File path errors are embarrassingly frequent. Package installation failures happen. Data type issues will haunt you.
Bottom line: importing Excel files into R isn't difficult. It just requires attention to detail. Get the prerequisites right, choose your method, and import away. Your data awaits.
Frequently Asked Questions
How to Handle Large XLSX Files Efficiently in R?
Large XLSX files in R? Not for the faint-hearted. Efficiency demands strategy.
Convert to CSV first—it's faster. Or use readxl package—beats xlsx hands down for speed. The xlsx2 function? Much quicker than standard read.xlsx().
Memory management matters. Import only what you need. Filter data beforehand. Preview files to spot issues. Nobody wants crashes mid-analysis.
Package choice makes or breaks performance. Openxlsx offers flexibility. Readxl delivers speed.
Can I Selectively Import Specific Sheets From an XLSX File?
Yes, selective importing is totally doable.
Using the readxl package, just specify which sheets you want with the "sheet" parameter. Like this:
'''r
library(readxl)
data <- read_excel("file.xlsx", sheet = "Sheet1")
'''
You can use sheet names or numbers.
Want to see what's available? The excel_sheets() function shows all sheets in your file.
Pretty handy when dealing with monster spreadsheets.
How to Troubleshoot Encoding Issues With Excel Files?
Encoding issues with Excel files? Total headache.
First, check for those pesky BOMs by opening files in a text editor. R users should specify encoding explicitly – "UTF-8-BOM" is your friend.
Excel loves using Windows-1252 encoding, causing weird characters. Try the readxl package instead of CSV exports.
Or save files as UTF-8 without BOM. Sometimes viewing hex data reveals hidden encoding gremlins.
No easy fix, just persistent troubleshooting.
What's the Fastest Package for Importing XLSX Files?
Readxl dominates the competition for importing xlsx files. No contest.
It's developed by Hadley Wickham and doesn't need external dependencies, making it blazing fast compared to alternatives. The xlsx package? Painfully slow with its Java dependency.
Openxlsx offers decent speed but can't match readxl's efficiency. Rio just piggybacks on other packages anyway.
For large datasets especially, readxl is the obvious choice. Speed matters.
Can R Modify and Save Changes Back to XLSX Files?
Yes, R can modify and save changes to xlsx files. Several packages make this possible. Openxlsx is popular—no Java required.
XLConnect offers more features but needs Java. The process? Read the file, manipulate data in R, then write it back.
Most packages preserve some formatting, but complex Excel features might get lost. Not perfect, but gets the job done. For simple edits, it's totally fine.