Threads are the smallest executable units within a process. They share memory resources but run independently, allowing computers to handle multiple tasks at once. Think of them as mini-workers in your computer's brain. User-level threads are managed by applications, while kernel-level threads are controlled by the operating system. They're why your browser doesn't freeze when loading multiple pages. Effective thread management prevents system crashes and boosts performance. The digital world would crawl without them.
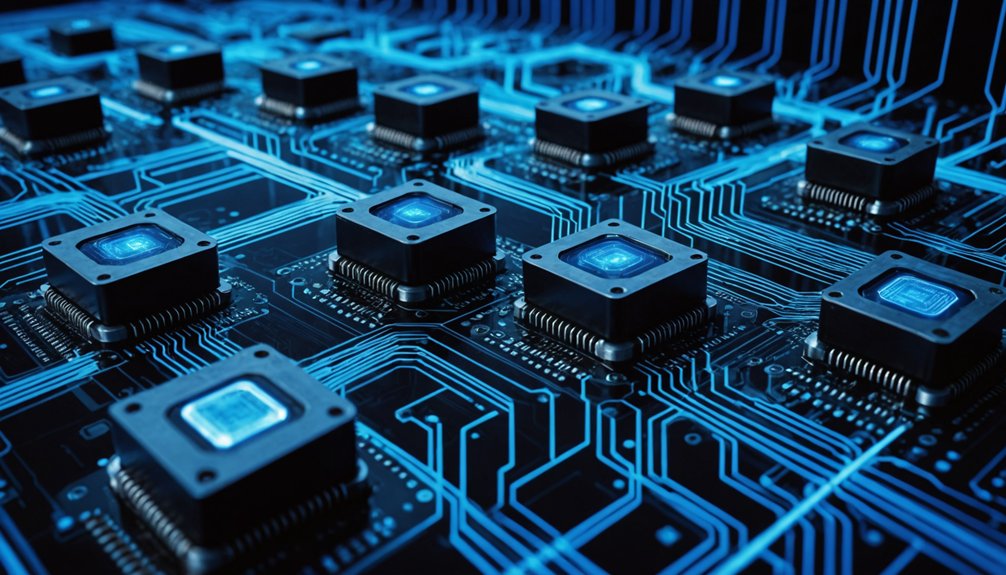
In the complex world of computing, threads form the backbone of modern software execution. These tiny powerhouses are the smallest sequence of instructions that a CPU can independently execute. Think of them as mini-workers in the vast factory of your computer, each handling their own task. They're sharing resources like memory with other threads in the same process, working together but separately. Neat, right?
Threads come in different flavors. User-level threads are managed by application code in languages like Java. Kernel-level threads are the operating system's domain. Some threads are cooperative, politely yielding control to others when they're done. Others are preemptive – they get interrupted mid-task when the scheduler decides their time is up. Then there's fibers, the DIY enthusiasts of the thread world, scheduling themselves. Similar to model training, threads require careful optimization to achieve peak performance. Understanding Big O notation helps developers optimize thread performance by analyzing algorithmic efficiency.
Multithreading is where things get interesting. It's like having multiple cooks in the kitchen instead of just one frantic chef. CPUs love this approach because it keeps them busy. Hyper-threading even divides physical cores into logical ones for extra multitasking goodness. Context switching between threads does create overhead – it's the cost of doing business. Threads enable CPUs to execute multiple tasks concurrently, significantly improving system performance and efficiency. Even with just a single CPU, effective scheduling creates an illusion of parallelism as the processor rapidly switches between different threads.
The benefits? Multitasking becomes less of a pain. Your interface stays responsive while heavy calculations happen in the background. System throughput increases. Latency decreases. Complex operations become manageable. It's a win-win-win-win-win situation.
Multithreading turns computing from a one-lane road into a superhighway of parallel productivity.
Of course, managing these threads isn't simple. Scheduling algorithms determine who gets CPU time. Priority scheduling puts important threads first. Thread pools reuse threads to avoid the overhead of creating new ones. Locks prevent chaos when multiple threads try to access the same resource.
Threads are everywhere in computing. They keep your browser tabs loading while you scroll Facebook. They handle multiple requests to web servers. They keep your mobile apps responsive. They manage database queries. They make your video games immersive. Without threads, computing would be a sequential bore. Who wants that?
Frequently Asked Questions
How Do Threads Differ From Processes?
Threads are lightweight. Processes aren't. Simple as that.
Threads share memory space within a process, making them faster to create and switch between. Processes? Completely isolated with separate memory spaces.
Multiple threads can exist in one process, sharing resources and code. When a thread crashes, it can take down the whole process. Processes remain unaffected by other failing processes.
Thread communication is efficient; process communication is more complex.
Can Too Many Threads Cause Performance Issues?
Yes, excessive threads absolutely cause performance issues.
Too many threads mean more context switching overhead. The CPU wastes time juggling threads instead of doing real work. Threads fight over limited resources like memory and cache. Imagine twenty people trying to use one bathroom—chaos!
Performance actually drops after a certain point. Thread management requires synchronization, which adds complexity. Finding the ideal thread count is essential and varies by system.
No free lunch here.
What Causes Thread Deadlock?
Thread deadlock happens when four conditions converge: mutual exclusion, hold-and-wait, no preemption, and circular wait.
It's a programming nightmare. Threads get stuck indefinitely, waiting for resources that other threads won't release.
Classic example? Thread A holds Resource 1 and needs Resource 2, while Thread B holds Resource 2 and needs Resource 1.
They're both trapped. Forever. The system freezes, memory gets wasted, and developers lose their minds debugging the mess.
How Are Threads Implemented Differently Across Operating Systems?
Operating systems handle threads differently.
Windows NT uses kernel-level threads for CPU-intensive tasks.
Solaris implements a two-tier model with both user and kernel threads.
Thread creation speed varies—Solaris creates threads faster than Windows.
Synchronization tools differ too; NT's critical sections outperform Solaris in some cases, while Solaris mutexes handle heavy synchronization better.
The implementation affects performance, scheduling, and resource management.
Pretty technical stuff, actually.
Are Threads Always Better Than Sequential Programming?
Threads aren't always superior to sequential programming. Sometimes they're overkill.
For simple tasks or systems with limited resources, the overhead of thread management can actually slow things down. Race conditions are a nightmare to debug. Sequential code is more straightforward—easier to understand, maintain, and predict.
On multi-core systems with independent tasks? Threads shine. But for real-time systems or applications with strict timing? Sequential might win.
Context matters.