Big O notation helps developers gauge how algorithms perform as data grows. Different complexity categories like O(1), O(n), and O(n²) reveal whether code will gracefully handle larger datasets or crash and burn. Sorting algorithms like merge sort cruise at O(n log n), while bubble sort crawls at O(n²) – yeah, big difference. Space complexity matters too since memory isn't infinite. Smart algorithm choices can mean the difference between lightning-fast apps and frustrated users throwing their devices. There's a whole world of optimization waiting to be explored.
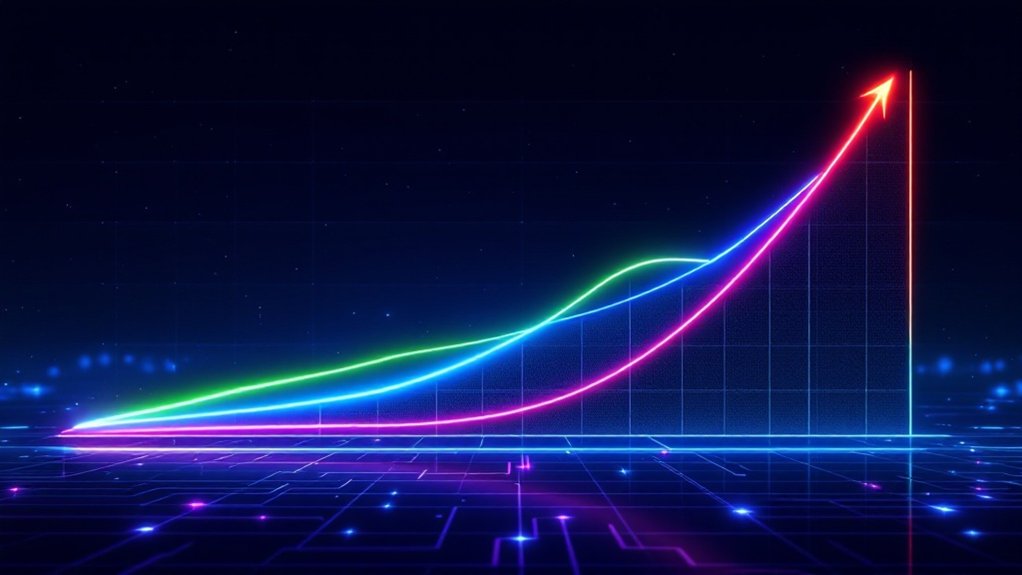
Every programmer's nightmare is watching their beautiful code crawl to a halt when the data gets big. That's where Big O notation comes in – the ultimate reality check for algorithmic efficiency. It's not just some fancy mathematical concept; it's the difference between your application running smoothly or crashing spectacularly when user counts skyrocket. The focus on worst-case scenarios provides crucial insights for real-world applications. Understanding how algorithms scale means examining their growth rates relative to input size.
Let's get real about complexity categories. Some algorithms are constant time O(1) – they're the speed demons that don't care how much data you throw at them. Others are linear O(n), taking their sweet time to process each item one by one. Then there's the sneaky logarithmic time O(log n), like binary search, cutting through data like a hot knife through butter. But watch out for those quadratic O(n²) monsters lurking in nested loops – they'll eat your processing power for breakfast. Like model training, the efficiency of your algorithm becomes increasingly critical as datasets grow larger.
Take sorting algorithms, for instance. Merge sort struts around with its O(n log n) complexity, while bubble sort stumbles along at O(n²). Sure, bubble sort might look simpler, but it's like bringing a butter knife to a sword fight when dealing with big data sets. Just as activation functions help neural networks process data efficiently, choosing the right sorting algorithm is crucial for optimal performance.
Quick sort tries to be clever with its average O(n log n), but it can face-plant into O(n²) territory if you're unlucky.
Space complexity is the silent killer that nobody talks about until the memory alerts start screaming. Some algorithms are memory misers, staying at O(1), while others gobble up space like there's no tomorrow, scaling linearly or worse with input size. And don't get started on those exponential space hogs – they'll have your system begging for mercy.
The real world doesn't care about theoretical perfection. Database queries need optimization, data structures need careful selection, and user experience hangs in the balance. Missing hidden loops or ignoring edge cases? That's a rookie mistake that'll come back to bite you when production servers start melting down.
Frequently Asked Questions
How Does Big O Notation Handle Multiple Input Variables?
Big O notation handles multiple variables by keeping all significant terms that contribute to growth rate.
No shortcuts here – if you can't prove one term dominates, they all stay. For instance, O(n²m + m²n) can't be simplified without knowing how n and m relate.
Variables interact, sometimes dramatically. Constants get dropped, but independent variables stick around unless there's a clear relationship between them.
It's that simple.
When Should I Prioritize Space Complexity Over Time Complexity?
Space complexity should take priority in memory-constrained environments like embedded systems and IoT devices. Period.
Real-time applications with strict memory limits can't afford bloated algorithms, no matter how fast they run.
Network-heavy applications benefit too – less memory means faster data transfer.
Sure, it might run slower, but when your device has the memory capacity of a potato, space efficiency wins.
What Are Practical Ways to Identify Big O Complexity in Existing Code?
Start by counting loops – they're dead giveaways. Single loops? O(n). Nested loops? Usually O(n²).
Look for recursion patterns and divide-and-conquer approaches.
Examine data structure operations like array access (O(1)) versus searching linked lists (O(n)).
Watch for built-in methods too – they're sneaky complexity bombs.
If the code splits data repeatedly, it's probably logarithmic O(log n).
Function calls within loops? Multiply those complexities.
How Do Nested Loops With Different Sizes Affect Big O Calculation?
Nested loops with different sizes multiply their individual complexities. A loop of size n containing a loop of size m yields O(n*m). Simple math, really.
When one loop size dominates (n >>> m), the larger one calls the shots. But watch out – if inner loop size depends on the outer loop's index, things get trickier.
Dynamic relationships between loops can create more complex patterns than simple multiplication.
Why Doesn't Big O Notation Consider Best-Case or Average-Case Scenarios?
Big O notation zeroes in on worst-case scenarios because that's what really matters for reliability.
Best-case situations? Too optimistic. Average cases? Too unpredictable and mathematically complex.