Converting Python dictionaries to JSON is straightforward with the 'json' module. Just import it, then use json.dumps) to transform your dictionary into a JSON string. Data types convert naturally – numbers stay numbers, booleans become true/false, None becomes null. For prettier output, add parameters like indent=4 or sort_keys=True. JSON's cross-language compatibility makes it perfect for APIs and web development. The rest of this guide unpacks trickier conversion scenarios.
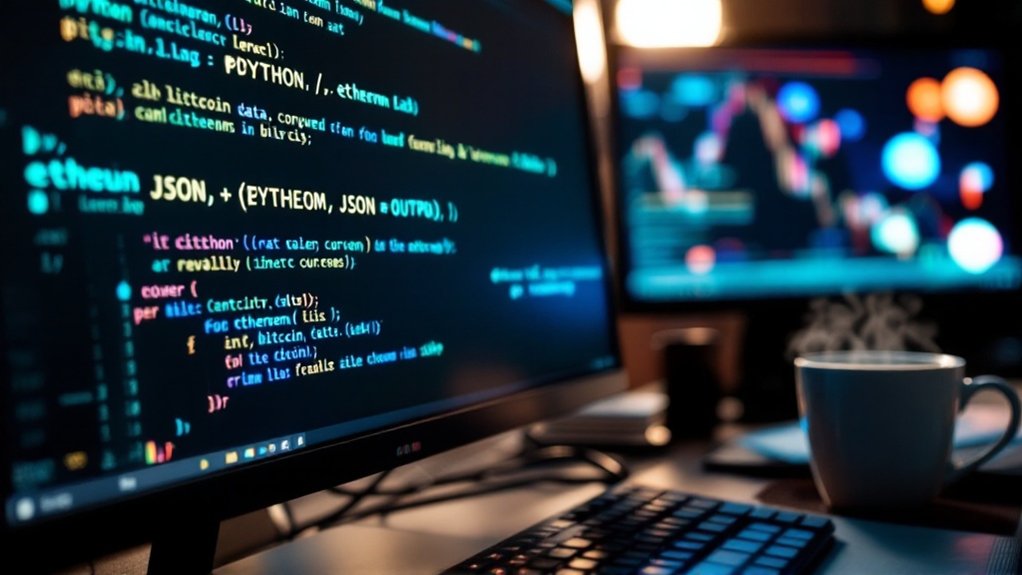
Transforming Python dictionaries into JSON isn't rocket science. It's a fundamental skill for anyone working with data in Python. The process hinges on the built-in 'json' module, which offers the handy 'json.dumps()' method. This little function takes your Python dictionary and spits out a JSON string. Simple as that.
Python dictionaries are natural candidates for JSON conversion. The structure matches perfectly – both use key-value pairs. When you convert, Python dicts become JSON objects, lists transform into arrays, and your standard Python types map directly to their JSON equivalents. Numbers stay numbers. Booleans become true/false. None values? They turn into null. No surprises there. Just like data preparation steps in machine learning, ensuring proper data formatting is crucial for successful conversion. Similar to how PCA analysis helps reduce data complexity, JSON conversion simplifies data exchange between systems.
The conversion syntax couldn't be more straightforward. Import the json module, call json.dumps(), and you're done. But wait – there's more to it than that. Want your JSON to look pretty? Use the 'indent' parameter. Need alphabetical order for your keys? Set 'sort_keys' to True. These little tweaks make a big difference when you're debugging or sharing data with humans.
JSON isn't just some techie obsession. It's everywhere. APIs use it constantly. Web developers can't live without it. It's the universal language of data exchange – the diplomat of the programming world. Unlike your cousin's obscure programming language, everyone understands JSON. This makes JSON perfect for easy data interchange between Python applications and web services.
Error handling matters too. Try converting a complex object with circular references and watch your code crash and burn. Smart developers anticipate these issues. For handling non-serializable data types like datetime objects, you'll need to create a custom serialization function.
The real beauty of JSON is its cross-language compatibility. Your Python application can spit out JSON that a JavaScript frontend happily consumes. Or store configuration in JSON files that multiple systems can read. It's like Esperanto, if anyone actually spoke Esperanto.
Bottom line: mastering Python-to-JSON conversion isn't optional anymore. It's table stakes for modern development. Learn it. Use it. Move on to harder problems.
Frequently Asked Questions
How Do I Pretty-Print JSON in Python?
For pretty-printing JSON in Python, there are three main approaches.
The cleanest way is using the json module with the indent parameter: 'json.dumps(data, indent=4)'. Simple and effective.
The pprint module works too, though it outputs Python format rather than strict JSON.
Command line fans can use: 'python -m json.tool file.json'.
Each method formats that jumbled JSON mess into something actually readable.
Pick your poison.
Can I Serialize Custom Python Objects to JSON?
Yes, custom Python objects can be serialized to JSON. By default, they'll throw a TypeError.
There are two main ways to fix this. First, add a method like 'to_dict()' that converts the object to a dictionary. Second, extend 'JSONEncoder' and override its 'default' method.
Both approaches let developers handle complex types like datetimes. Some developers wish Python had a built-in '__json__' method, but it doesn't.
It's not hard, just needs extra code.
How to Handle Date and Time Objects in JSON?
Handling date and time in JSON isn't straightforward. JSON doesn't natively support date objects. The go-to solution? ISO 8601 format (like "2023-11-02T15:30:45Z"). It's universal. Period.
For Python specifically, you'll need to convert datetime objects to strings before serialization. The json module can't handle them directly.
Many developers store dates as UTC and include timezone info. Smart move. Using Unix timestamps is another option – compact but less readable. Consistency is key here.
What's the Difference Between Json.Dump() and Json.Dumps()?
The difference is simple. json.dump) writes straight to a file while json.dumps) returns a string. That's it. Same parameters, same functionality otherwise.
You need to save JSON data? Use dump() with a file object. Need a JSON string to pass around in your code? That's dumps().
They're basically twins – one's just more into writing things down. Both handle the conversion from Python objects to JSON format.
How Do I Handle Unicode Characters in JSON?
To handle Unicode characters in JSON, developers should use UTF-8 encoding. It's the gold standard. Period.
When working with Python, set 'ensure_ascii=False' in json.dumps() to preserve those fancy characters instead of converting them to uXXXX escape sequences. JSON naturally supports Unicode—that's the whole point.
For database storage, stick with UTF-8 compatible formats. Some systems might choke on certain characters, so proper encoding declaration is essential.
Test your JSON with emoji. Seriously.