Python offers multiple ways to merge dictionaries. The classic approach uses dict1.update(dict2), which modifies the original. Modern methods include the unpacking operator {dict1, dict2} (Python 3.5+) and the merge operator dict1 | dict2 (Python 3.9+). For in-place updates, try dict1 |= dict2. When keys overlap, later dictionaries overwrite earlier values. Advanced options include collections.ChainMap and dictionary comprehensions for more complex merging scenarios. These techniques handle everything from simple combinations to intricate data structures.
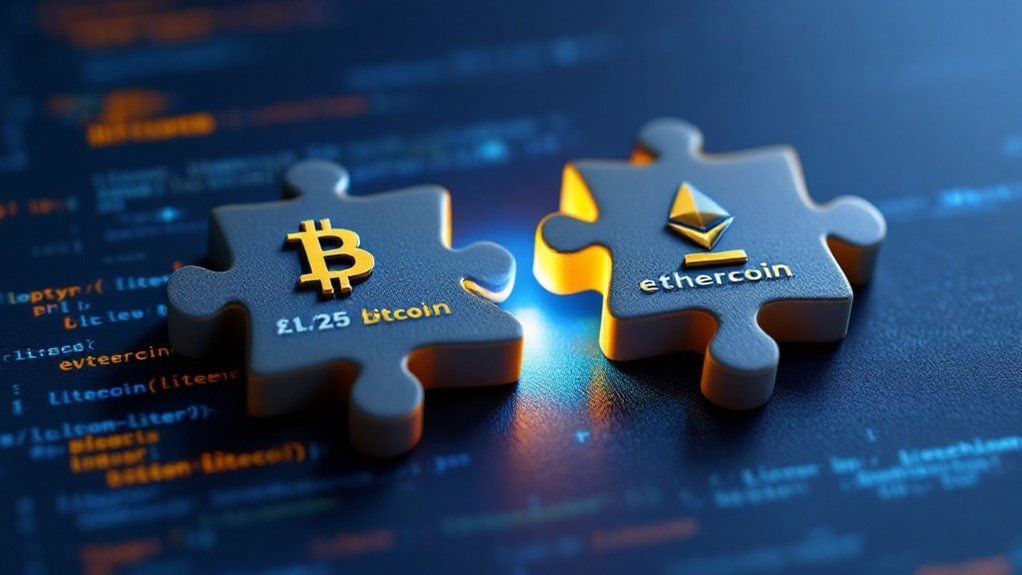
Juggling multiple dictionaries in Python? It happens to the best of us. Data scattered across different dictionaries. Messy. Inefficient. But Python offers multiple ways to combine them into a single, coherent structure.
The classic approach uses the 'update()' method. Been around forever. Works in all Python versions. Simple syntax: just call 'update()' on your first dictionary with the second as an argument. Like this: 'dict1.update(dict2)'. The catch? It modifies your original dictionary. If you need both dictionaries intact, look elsewhere. The direct assignment approach also works for basic dictionary modifications.
Python 3.5 introduced the unpacking operator. Game changer. Using '{dict1, dict2}' creates a new merged dictionary without touching the originals. Clean. Elegant. The original dictionaries remain untouched, which is often exactly what you want.
Then Python 3.9 came along with the merge operator '|'). Even better. Just write 'dict1 | dict2'. Boom. New dictionary with everything combined. For in-place updates, there's also the update operator ('|='): 'dict1 |= dict2'. Straightforward. No nonsense. The square brackets syntax provides direct access to individual values after merging.
Need something more advanced? The 'itertools.chain()' method lets you merge dictionaries into a single iterable. Import it first, then use 'dict(chain(dict1.items(), dict2.items()))'. A bit verbose, but gets the job done.
There's also 'collections.ChainMap()'. Different approach entirely. It creates a view of multiple dictionaries without copying data. Any changes to the originals show up in the ChainMap. Efficient, but with different semantics.
For complex scenarios, dictionary comprehensions offer fine-grained control. You can filter keys, handle nested structures, even implement conditional merging logic. Dictionary comprehensions are particularly useful when data integrity needs to be maintained during the merging process. Not for beginners, though.
Remember: with overlapping keys, later dictionaries win. The value from the last dictionary overwrites previous ones. That's just how it works. Deal with it.
Choose your method based on Python version and specific needs. They all merge dictionaries. They just do it differently. While most examples show merging two dictionaries, you can extend these methods to combine multiple dictionaries simultaneously for more complex data structures.
Frequently Asked Questions
How Do I Handle Key Conflicts When Merging Dictionaries?
When dictionaries collide, someone's gotta lose. By default, the second dictionary wins these key battles. Most methods (update(), unpacking with **, or the | operator) simply overwrite existing values. No negotiation.
For custom solutions, developers can implement loops to handle conflicts their way. Maybe average the values? Combine them? Keep both? The choice is yours.
ChainMap is the rebel—it favors the first dictionary instead. Performance varies, but readability matters more for most code.
Can I Merge Dictionaries With Different Value Types?
Yes, Python doesn't care about value types when merging dictionaries. Integers, strings, lists, whatever—they'll all happily coexist in your merged result.
Different data types don't transform during merging; they just get combined as-is. When dict1 has {"a": 1} and dict2 has {"b": "hello"}, the merged dictionary simply contains both entries.
No type conversion, no fuss. Just be careful with operations that require comparable types afterward.
Does Merging Dictionaries Affect the Original Dictionaries?
Merging dictionaries can affect originals, but it depends on your method.
Using 'update()' modifies the first dictionary—original gone forever. Shocker.
The '' operator and '|' merge operator (Python 3.9+) create new dictionaries** instead, leaving originals untouched. Smart move.
'ChainMap()' and 'chain()' also preserve the originals.
Bottom line: if you want to keep your dictionaries intact, avoid 'update()' or make copies first. Not rocket science, just basic Python behavior.
What's the Performance Impact of Merging Large Dictionaries?
Merging large dictionaries can be a real memory hog. Creating new dictionaries with unpacking operators (like {a, b}) demands more resources than in-place methods.
Update() and |= operators modify existing dictionaries—less memory, more speed. For truly massive data, itertools.chain shines without creating intermediate objects.
Python version matters too; 3.9's merge operator (|) offers cleaner syntax.
Bottom line? Choose your method based on your memory constraints. Memory isn't infinite, folks.
How Can I Merge Nested Dictionaries Recursively?
To merge nested dictionaries recursively, developers need a custom function that checks if values are dictionaries themselves.
When they are, it dives deeper. Simple as that. Here's the general approach:
'''python
def recursive_merge(dict1, dict2):
for key, value in dict2.items():
if key in dict1 and isinstance(dict1[key], dict) and isinstance(value, dict):
recursive_merge(dict1[key], value)
else:
dict1[key] = value
'''
Not rocket science. Just recursion doing its thing.