Numpy's linalg.norm) calculates vector or matrix magnitude. It handles multiple norm types: L1 (absolute sum), L2 (Euclidean), infinity (maximum value), and more. The function's syntax includes parameters for order, axis specification, and dimension retention. Scientists rely on it for everything from data preprocessing to signal analysis. It's not just math—it's the backbone of countless computational solutions. The deeper you go with this function, the more computational doors swing open.
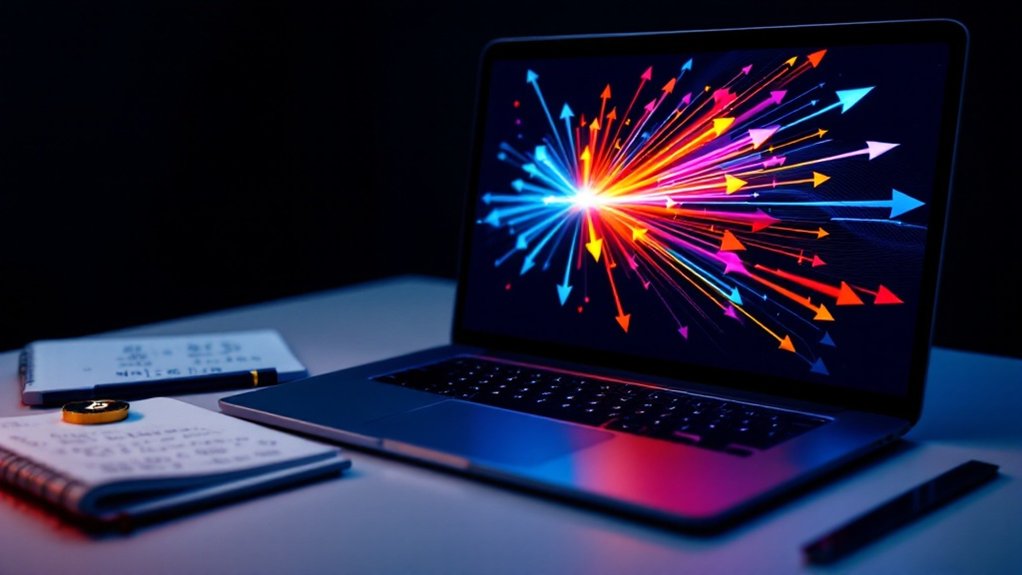
Linear algebra—it's the backbone of countless computational tasks. When working with vectors and matrices in Python, NumPy's linalg.norm) function is a powerhouse tool that shouldn't be overlooked. This function calculates the norm of a matrix or vector, which is fundamentally a measure of its "size" or "magnitude." Pretty straightforward, right? Well, there's more to it.
The syntax is clean: numpy.linalg.norm(x, ord=None, axis=None, keepdims=False). It takes an input array, an order parameter, an axis specification, and a boolean to keep dimensions. Nothing fancy, just practical. Much like data validation in data preparation, proper syntax ensures accurate and reliable results.
Norm types matter. There's the L1 norm (sum of absolute values), L2 norm (the classic Euclidean distance), infinity norm (maximum absolute value), and others like Frobenius and nuclear norms for matrices. Each serves a different purpose. Choose wisely. Like data preparation steps, selecting the right norm type is crucial for achieving accurate results.
Your choice of norm defines how you measure magnitude in your computational space. Different problems demand different metrics.
The axis parameter is surprisingly useful. Want to find norms along columns? Use axis=0. Rows? Try axis=1. No axis specified? You'll get a single scalar representing the entire array's norm. It's versatile like that.
Matrix norms require special attention. By default, you'll get the Frobenius norm—essentially the Euclidean norm applied to the entire matrix as if it were flattened. But sometimes you need the nuclear norm (sum of singular values) or the infinity norm (maximum row sum). The function handles all these cases. You can also compute the minimum absolute row sum by setting ord=-np.inf in your function call.
In practice, norms pop up everywhere. They're vital for data preprocessing in machine learning, solving systems of linear equations, analyzing signals, and defining constraints in optimization problems. Scientists and engineers use them daily without a second thought.
The beauty of np.linalg.norm() lies in its simplicity and flexibility. One function, multiple norm types, various dimensional options. Setting the keepdims parameter to True ensures that the dimensions with size one are retained in the output, which can be critical for maintaining array compatibility in subsequent calculations. It's the kind of tool that makes computational work bearable. Sometimes even enjoyable. Who would've thought?
Frequently Asked Questions
How Does Numpy.Linalg.Norm() Perform With Sparse Matrices?
NumPy's linalg.norm() doesn't handle sparse matrices efficiently. At all. It treats them as dense matrices, which defeats the whole purpose. Memory usage skyrockets. Computations crawl.
For sparse matrices, SciPy's sparse.linalg.norm) is the way to go. It's specifically designed for sparse data structures. Faster. More memory-efficient. Handles various norm types too – Frobenius, infinity, the works.
Bottom line: stick with SciPy for sparse matrices. NumPy just wasn't built for this.
Can Numpy.Linalg.Norm() Calculate Matrix Norms on GPU?
NumPy's linalg.norm) can't calculate matrix norms on GPU. Period. It's a CPU-only function—no ifs, ands, or buts about it.
For GPU-accelerated norm calculations, you'll need to look elsewhere. CuPy, PyTorch, or TensorFlow are your best bets. They offer similar functionality with that sweet GPU acceleration.
Want those matrix norms to fly? Gotta ditch vanilla NumPy and embrace the GPU-friendly alternatives. That's just how it works.
What Are Performance Differences Between Numpy.Linalg.Norm() and Scipy.Linalg.Norm()?
Performance differences between numpy.linalg.norm() and scipy.linalg.norm() aren't one-size-fits-all.
SciPy often wins. Why? Always compiled with BLAS/LAPACK support. NumPy? Not guaranteed.
SciPy may execute faster for large matrices and complex operations.
But! NumPy has that handy 'axis' parameter that SciPy lacks. Trade-offs exist.
Real-world impact depends on data size, norm type, and specific hardware.
Need absolute certainty? Benchmark your specific use case.
How Does Numpy.Linalg.Norm() Handle Nan Values?
NumPy's linalg.norm() doesn't play nice with NaN values. Simple as that. If there's even one NaN in your array, the entire norm result becomes NaN. No exceptions, no special options to ignore them.
This NaN propagation happens across all norm orders—L2, L1, whatever.
Want to avoid this headache? You'll need to preprocess your data first. Replace those NaNs or mask them out.
Standard math rules, folks. Harsh but consistent.
Are There Faster Alternatives for Large-Scale Norm Calculations?
For large-scale norm calculations, several faster alternatives exist. JAX shines with GPU acceleration—blazing fast.
Numba compiles NumPy code for serious speed boosts. Scipy sometimes outperforms the original. Cython? Even better if you're willing to get your hands dirty with C-like code.
For truly massive datasets, distributed computing frameworks like Dask or Apache Spark divide and conquer.
Data type optimization matters too—float32 instead of float64 can work wonders. Memory efficiency isn't just nice, it's necessary.