Updating Python dictionaries is dead simple. Use the 'update()' method to modify dictionaries in-place: 'my_dict.update(new_dict)'. Boom—original dictionary changed, no new object created. It merges key-value pairs, overwrites existing keys without warning. For more control, traditional assignment works too: 'my_dict[key] = value'. Need conditional updates? Add an if-statement to check for existing keys first. Careful though—accidentally overwritten values don't come back. The deeper techniques await those who venture beyond the basics.
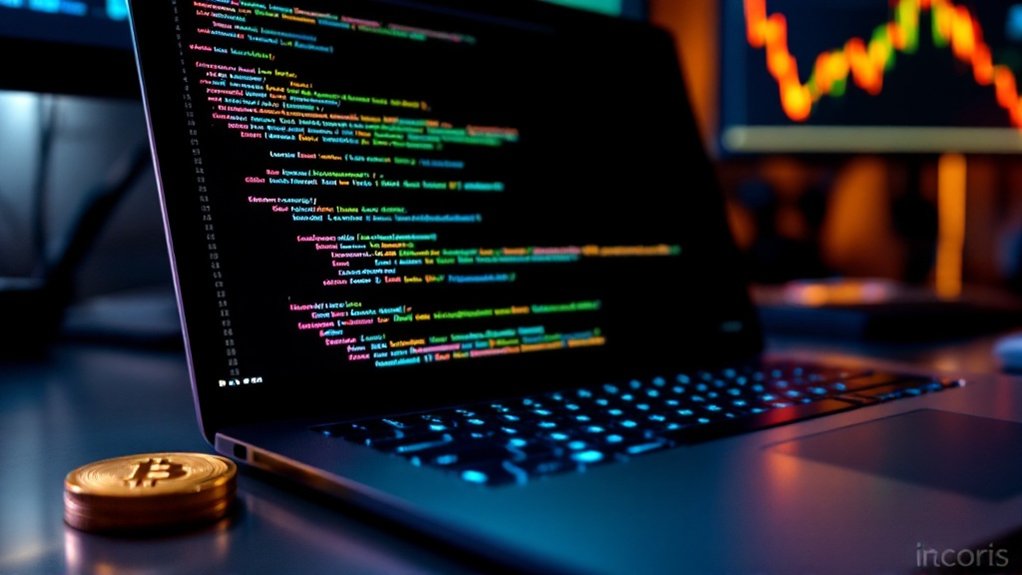
Dictionaries in Python pack a punch when it comes to storing and retrieving data. They're flexible, efficient, and downright useful for organizing information. But what happens when you need to modify that data? That's where updating comes in.
The 'update()' method is your go-to solution. Simple syntax: 'dict.update(iterable)'. No rocket science here. This method takes key-value pairs from another dictionary or iterable and adds them to your original dictionary. The beauty? It happens in-place. No fancy return values—just 'None' and a modified dictionary. Converting dictionaries to JSON strings enables seamless data serialization across platforms. The square bracket notation provides direct access to modify individual dictionary values.
Update dictionaries with a single method—no muss, no fuss. It's Python's elegant solution to data modification.
Got duplicate keys? Too bad. The original values get overwritten. No questions asked. Say you have a 'books' dictionary and updatethe price of 'Fluent Python'—the old price is history. Gone. But don't worry, this doesn't stop new keys from being added. The dictionary just grows with both updated and fresh entries.
For loops offer another approach. More verbose? Yes. More control? Absolutely. You can iterate through another dictionary's items using '.items()' or '.keys()' methods, then assign new values with the humble equals sign. When dealing with multiple dictionaries like 'books' and 'more_books', for loops iterate through each key systematically. It's wordier than 'update()', but sometimes clarity trumps brevity.
Want to be cautious? Throw in some conditional logic. Check if a key exists before updating with an 'if key not in dict' statement. This prevents accidental overwrites—crucial when preserving existing data matters. Real-world applications use this constantly.
Watch out for common mistakes. The 'update()' method doesn't care about your precious data—it'll overwrite existing values without a second thought. Be intentional about what you're updating. When incrementing values of existing keys, you can use the increment by one pattern as shown in the dictionary_update function.
Using 'update()' reduces code complexity. It's particularly handy when merging multiple dictionaries. Why write twenty lines when you can write two? Efficiency matters. Python gives you options. Choose wisely.
Remember: dictionaries aren't just containers. They're powerful tools that, when updated properly, make data management a breeze. Not rocket science. Just good programming.
Frequently Asked Questions
Can I Use Dictionary Comprehension to Update Dictionaries?
Dictionary comprehensions can't directly update existing dictionaries – they create new ones instead. That's just how they work.
Developers can use a two-step process though: create a new dictionary with comprehension, then assign it back to the original variable. It's an indirect approach, but it works.
For direct updates, the update() method is better. Dictionary comprehensions shine at transformations and filtering, not modifications.
Sometimes the obvious solution isn't available. Deal with it.
How Do I Update Nested Dictionaries in Python?
Updating nested dictionaries in Python? Not rocket science. Developers can use recursive functions to dig through multiple levels, or leverage the built-in update() method for specific nesting levels.
For missing keys, setdefault() or defaultdict are lifesavers. Want to avoid messing up the original? Deep copy it first. The deeper the nesting, the slower the updates.
Some use dictionary comprehensions for concise updates. Error handling is essential, obviously. Nobody likes KeyErrors popping up everywhere.
Is Dictionary Updating Thread-Safe in Python?
Simple dictionary operations in Python aren't fully thread-safe. Thanks to the GIL, basic updates won't crash your program, but complex operations can still cause race conditions.
One thread might read while another writes, giving unpredictable results. The GIL isn't a magical safety net. For truly thread-safe dictionary operations, developers should use locks or specialized thread-safe data structures.
No shortcuts here – concurrent programming requires proper synchronization.
What's the Time Complexity of Dictionary Update Operations?
Dictionary update operations in Python typically run in O(n) time complexity, where n is the number of elements being updated. Simple enough.
Hash collisions can mess things up though, potentially degrading performance to O(n*m) in worst cases, with m being collision count. For most real-world scenarios? The average O(n) holds.
Python's implementation is pretty efficient. Each key-value pair gets processed individually, so naturally, more items mean more processing time. Nothing mysterious there.
How Can I Track Changes When Updating Dictionaries?
To track dictionary changes, developers can implement a simple logging system. Print statements work fine for basic needs.
More robust? Use Python's logging module. Creating a change history dictionary is smart—it stores all modifications with timestamps for accountability.
Some even build custom wrapper classes that automatically log operations. Audit trails aren't just for paranoid programmers. They're essential for debugging complex applications.
Want to know who broke what when? Proper change tracking answers that question. No more mystery crashes.