Adding rows to a DataFrame in Python can be done several ways. The DataFrame.loc[] method modifies the existing DataFrame directly with df.loc[len(df)] = new_row. Pandas.concat) joins DataFrames together, creating a new one – useful for preserving originals. The append() method still works but it's deprecated, so avoid it. Dictionary-based addition works great for simple operations, while list comprehension handles multiple rows efficiently. The right technique depends on your specific needs. The deeper techniques reveal themselves to those who stick around.
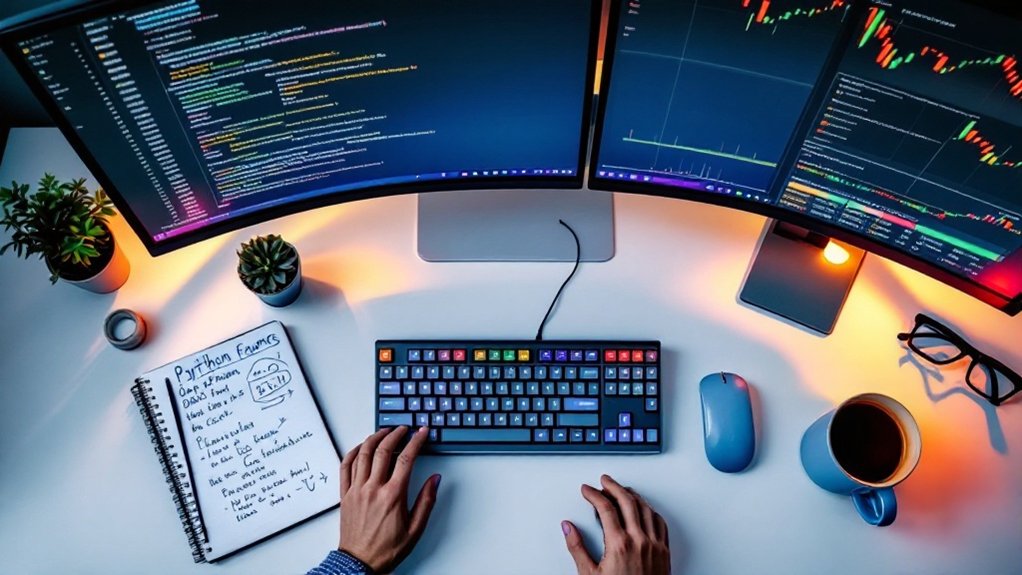
Adding rows to a DataFrame is a common task for Python data analysts. They need to understand multiple methods to handle this efficiently. Not all approaches are created equal. Some modify the original DataFrame, while others create new ones.
The DataFrame.loc[] method offers direct modification. It accesses the DataFrame by label and adds rows at specified indexes. Simple and straightforward. Just create a dictionary with column names as keys, then use df.loc[len(df)] = dict_row. Done. The DataFrame changes in place—no new object needed. Starting with initial data assessment helps ensure your DataFrame is ready for modifications.
Using df.loc[len(df)] = your_dict is the easiest way to add rows—simple, direct, and modifies in place.
Pandas.concat() provides more flexibility. It joins DataFrames together and preserves the original. Want to add multiple rows efficiently? This is your method. It creates a new DataFrame object rather than modifying the existing one. Specify axis=0 for row concatenation. There are plenty of options for handling indexes too. You can use ignore_index=True parameter to reset the index of the resulting DataFrame. Similar to how Beautiful Soup parses HTML elements, concat() systematically combines data structures.
DataFrame.append() used to be popular. Not anymore. It's been deprecated since pandas 1.4.0. Time to move on. It still works (for now) but don't get attached.
Creating a new DataFrame with row data and concatenating it offers maximum flexibility. Perfect for adding multiple rows simultaneously. Create your data structure, make a small DataFrame, then concat it with the original. Reset the index afterward if needed. When using a specific index, you can append with custom index names for better organization and reference.
Dictionary-based row addition is clean and efficient. Match column names exactly or face the consequences. It's particularly good for single row operations.
List comprehension methods shine for batch operations. Create dictionaries for your rows, throw them in a list, make a DataFrame, and concatenate. Column order stays intact.
Performance matters. Iterative appending is computational suicide for large datasets. Batch additions win every time. For massive datasets, consider chunking or building lists first, then making one concatenation at the end. Your CPU will thank you.
Frequently Asked Questions
How Do I Efficiently Add Multiple Rows at Once?
To add multiple rows efficiently, developers should use the concat() function. It's faster than append) – way faster.
Create a DataFrame with the new rows first, then concatenate it with the original. Like this:
'''python
new_rows = pd.DataFrame([row1_dict, row2_dict, row3_dict])
df = pd.concat([df, new_rows], ignore_index=True)
'''
This approach beats adding rows one by one. Performance matters, especially with larger datasets.
Can I Add Rows With Different Column Names?
Yes, rows with different column names can be added to a DataFrame using pd.concat).
It handles mismatched columns automatically. With join='outer' (default), it keeps all columns and fills missing values with NaN.
With join='inner', it keeps only shared columns. Alternatively, a dictionary with the new row data can be converted to a DataFrame first, then concatenated. Column alignment happens automatically.
Sometimes messy, but gets the job done.
How to Handle Index Conflicts When Adding Rows?
Index conflicts happen. Deal with them.
When adding rows to DataFrames, you've got options. Use ignore_index=True to auto-assign new integers. Reset the index after adding rows for clean sequencing. Create custom indexes with timestamps or UUIDs.
For existing duplicates, filter with df[~df.index.duplicated()]. Each method has trade-offs. Pick what makes sense for your data. No perfect solution here—just practical choices.
What's the Performance Impact of Repeatedly Adding Rows?
Repeatedly adding rows to a DataFrame? Performance nightmare. Each append creates an entirely new DataFrame. Memory usage skyrockets linearly. Execution time? Even worse—grows quadratically with row count.
Fine for tiny datasets, absolute disaster for anything substantial.
Loop appending can bring systems to their knees. Smart developers avoid it. They use pd.concat() or create lists first, then convert.
Append is convenient, sure, but the performance hit? Brutal.
How to Add Rows Conditionally Based on Existing Data?
Conditional row addition can be done several ways. Use df.loc[condition] for simple filtering with a single new row.
For complex logic, apply() with a custom function works but can be slow. Concatenation with pd.concat) is better for adding multiple rows at once.
The mask() method offers a hybrid approach. Each method has trade-offs.
Repeated additions kill performance, so batch operations are always smarter.