Time complexity calculation requires analyzing an algorithm's basic operations in relation to input size. Count operations within loops (single loops = linear, nested = polynomial), examine recursion depth, and assess conditional branches. Always consider the worst-case scenario. After calculating, simplify by dropping constants and lower-order terms to express in Big O notation. It's not rocket science, but precision matters. The difference between O(n) and O(n²) might just save your app from becoming unusably slow.
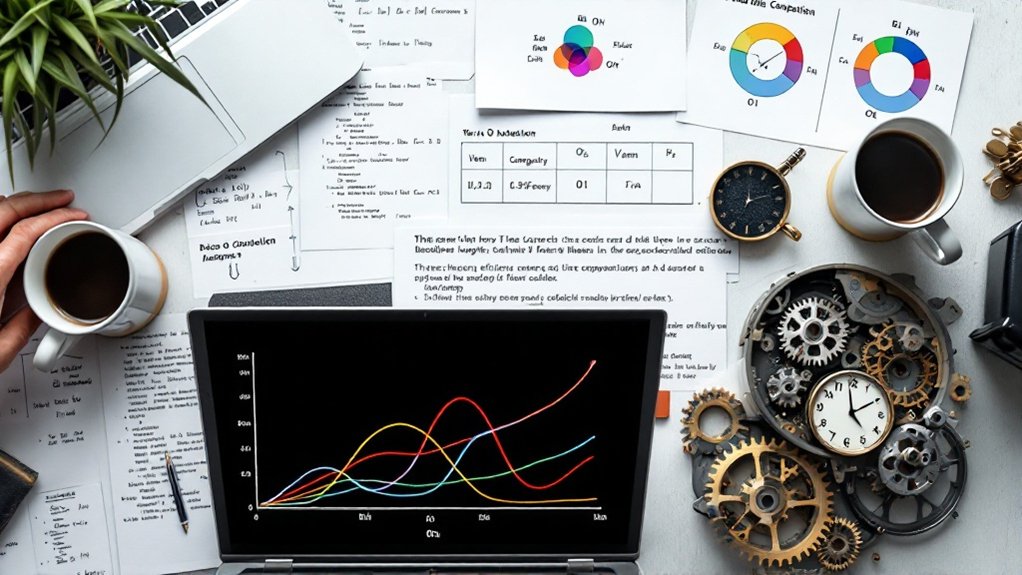
Mastering time complexity analysis is essential for any serious programmer. It's not just academic fluff—it's the difference between code that breezes through millions of operations and code that crashes spectacularly. The process starts with identifying basic operations: arithmetic calculations, comparisons, assignments. Count them. They matter. Every single one.
Input size determination comes next. Is your algorithm processing an array? Count its length. A string? Its characters matter. Multiple inputs? Account for all of them. This is your "n" or whatever variable you want to use. It's the foundation of everything that follows. Understanding time complexity categories helps classify algorithms from constant to factorial efficiency.
Your algorithm's power depends on its input. Define what "n" means—arrays, strings, matrices—before you analyze anything else.
Loops will make or break your algorithm. Nested loops? You're probably looking at polynomial time. A single loop iterating through all elements is linear. Simple math, really. But watch those variable bounds and step sizes—they can be sneaky. Remember that time complexity focuses on worst-case scenarios when evaluating algorithm performance.
Recursive functions require special attention. Find the base case first. Then count those recursive calls. How deep does this rabbit hole go? The recursion depth multiplied by work per call gives you complexity. Some problems actually become elegant with recursion. Others become disasters. Know the difference. Just like model training in machine learning, the process requires careful monitoring and evaluation.
Don't forget conditional statements. If-else branches and switch-cases create different execution paths. The worst-case scenario is what matters here. Always. No exceptions.
Now for the real work: calculate overall complexity. Sequential operations? Add them up. Nested operations? Multiply them. For conditionals, take the maximum. Apply the Master Theorem when facing divide-and-conquer situations. It's a lifesaver.
Final step: simplify. Drop those lower-order terms—they're dead weight. Remove constant coefficients. Nobody cares if your algorithm runs in 5n² or n² time. It's still quadratic. Keep only what dominates as input grows. That's your Big O notation. This approach ensures that your algorithm analysis remains relevant as hardware advancements continue to evolve, focusing on scalability rather than implementation-specific details.
Time complexity isn't magic. It's methodical analysis anyone can learn. Even you.
Frequently Asked Questions
Is Space Complexity Equally Important as Time Complexity?
Space complexity isn't always equal to time complexity. Sometimes it matters more. Sometimes less. Depends entirely on the scenario.
Big data applications? Memory constraints are brutal. Mobile apps? Every byte counts.
But for many algorithms, time efficiency takes priority—users hate waiting.
The reality? Both matter. Smart developers consider trade-offs between the two. One might be sacrificed for the other. Context is everything.
When Should I Prioritize Readability Over Optimal Time Complexity?
Developers should prioritize readability over ideal time complexity when the code isn't in a performance-critical path. For most business applications, readability wins. Period.
Only optimize after profiling identifies actual bottlenecks. Long-term maintenance costs usually outweigh minor performance gains. Teams share code. Future-you will thank present-you for clear logic.
Besides, modern hardware makes many optimizations irrelevant. The exception? Real-time systems, gaming, and high-frequency trading. Those milliseconds matter.
How Do Different Hardware Architectures Affect Practical Time Complexity?
Hardware architectures fundamentally transform theoretical time complexities into real-world performance.
Multi-core processors make O(n) algorithms faster through parallelization.
Memory hierarchies? They're essential. An O(1) lookup becomes painfully slow with cache misses.
GPUs demolish certain O(n²) tasks that would cripple CPUs.
Specialized hardware can even make "exponential" algorithms practical.
Big-O analysis provides a foundation, but hardware determines what actually runs quickly.
Theory, meet reality.
Can Machine Learning Predict Algorithm Performance Better Than Big O?
Machine learning can predict algorithm performance better than Big O in specific contexts.
It captures nuanced behavior with real-world inputs and hardware specificities. Big O strips away details—ML embraces them.
But there's a catch. ML needs tons of training data and struggles with new algorithms.
Smart developers use both: Big O for theoretical bounds, ML for practical performance estimates. They're complementary tools, not competitors.
How Do Functional Programming Paradigms Impact Complexity Analysis?
Functional programming changes the complexity game.
Immutable data structures mean operations create new copies—often logarithmic instead of constant time. Recursion replaces loops, making analysis rely on recurrence relations. No state changes make reasoning easier, but space complexity typically increases.
Persistent data structures behave differently. The good news? Referential transparency and lack of side effects make proofs more straightforward. The bad? Higher-order functions can obscure what's actually happening under the hood.