Creating a Python chatbot requires a few key steps. First, set up a development environment with Python and a virtual environment. Install necessary libraries like ChatterBot and NLTK for natural language processing. Create a chatbot instance, then train it with conversation examples using pre-built corpus or custom data. More advanced applications can leverage frameworks like Rasa for complex conversations. Despite limitations, chatbots can handle basic customer service tasks when properly trained. The journey from simple responses to sophisticated conversational AI awaits.
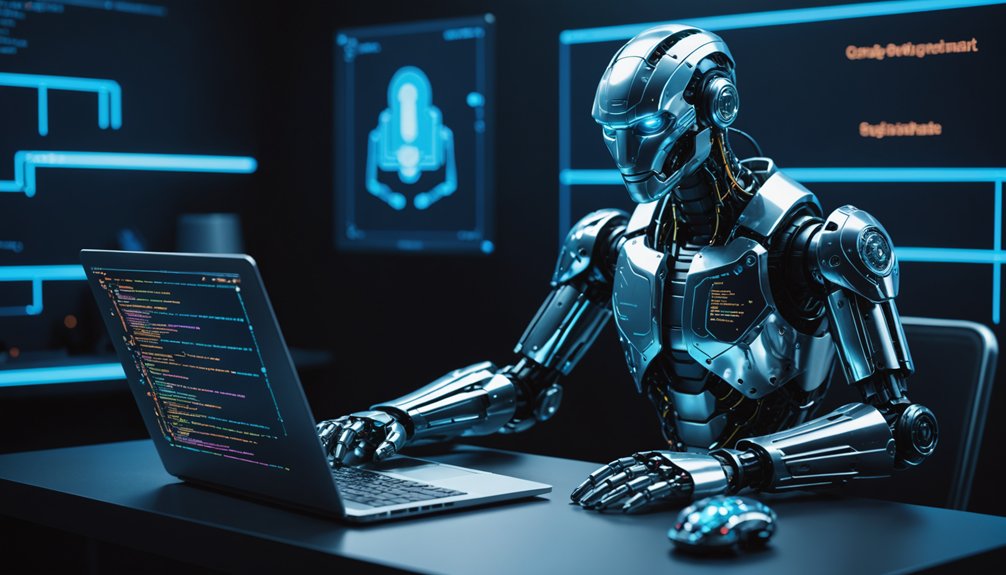
Creating a Python chatbot isn't rocket science—it's actually pretty straightforward if you follow the right steps. The process begins with setting up your development environment. You'll need Python installed on your system—duh. It's the foundation of any chatbot project thanks to its simplicity and powerful libraries.
Next, create a virtual environment using 'venv'. This keeps your project dependencies neat and tidy, away from other Python projects that might mess things up. Like any machine learning model, your chatbot needs clear objectives and well-defined problems before development begins.
Keep your Python projects isolated with a virtual environment—like giving each one its own clean room to play in.
Time to install some libraries. You can't build a decent chatbot without them. ChatterBot is popular for a reason—it's easy to use and language independent. Just type 'pip install chatterbot' and watch the magic happen. You'll also need 'nltk' for natural language processing. These packages do the heavy lifting when it comes to understanding human speech. Trust me, you don't want to code that from scratch.
Once everything's installed, you can create your chatbot instance. It's as simple as calling 'ChatBot('ChatbotName')'. Seriously, that's it. But a chatbot without training is like a car without gas—useless. You'll need to feed it conversation examples using ChatterBot Corpus or custom data. The bot uses algorithms like TF-IDF and cosine similarity to match user inputs with appropriate responses. Pretty clever for a bunch of code. Modern chatbots often utilize Transformer architecture for enhanced language understanding and generation capabilities.
For more advanced applications, frameworks like Rasa offer intent-based conversational flows. They're more complex but way more powerful. Storage adapters like SQL help store conversation data in a structured format. This means your bot can actually learn from past conversations. Not bad.
Python chatbots aren't perfect. They'll make mistakes and sometimes completely miss the point of a question. But they're getting better. With proper setup and training, they can handle basic customer service tasks or just chat about the weather. Not bad for a few hundred lines of code. You can also integrate your bot with Flask to provide a web interface for users to interact with your chatbot more easily. These chatbots rely heavily on Natural Language Processing to understand and respond to user inquiries appropriately.
Frequently Asked Questions
How Much Computing Power Is Needed for a Chatbot?
Computing power needs for chatbots vary wildly.
Simple rule-based bots? Run 'em on a potato. Advanced AI models? That's another story. Large language models demand serious hardware—GPUs with 10-24GB VRAM, substantial CPU, memory, and energy.
Training these beasts can gobble up 10 GWh of electricity. Crazy, right?
Local deployment requires optimization tricks like quantization to squeeze performance from limited resources. It's a balancing act of complexity versus hardware constraints.
Can Chatbots Integrate With Social Media Platforms?
Yes, chatbots absolutely integrate with social media platforms. They connect through APIs to Facebook, Instagram, WhatsApp, and more. Pretty handy stuff.
These integrations enable auto-responses to story reactions and platform-specific features. Messaging APIs handle the sending and receiving of messages. Developers can customize chat widgets to match each platform's aesthetic.
Rule-based, FAQ, and conversational AI bots all function across social channels. Integration's a no-brainer for businesses wanting 24/7 customer service.
What Privacy Concerns Arise When Deploying Chatbots?
Chatbots raise serious privacy red flags. They collect massive amounts of personal data—sometimes without users even knowing.
Encryption? Often lacking. Third-party sharing? You bet. Most users have zero idea what happens with their information after hitting "send."
GDPR and CCPA regulations try to rein this in, but compliance is spotty at best. Data breaches happen.
And that targeted advertising based on your conversations? Creepy, yet totally common.
How to Handle Multilingual Conversations in Python Chatbots?
Handling multilingual chats in Python? Not rocket science.
Developers typically integrate language detection tools like Google's CLD2 or Langid.py first. Then they implement NLP libraries—NLTK or spaCy work well.
Translation APIs handle the heavy lifting. Some use frameworks like LangChain with LLMs for real-time responses. The smart ones build scalable architecture from the start.
Testing across languages? Absolutely critical. Automated language detection saves users the hassle of manual selection.
What Metrics Best Measure Chatbot Effectiveness?
Chatbot effectiveness boils down to a few key metrics.
Resolution rates show if users get answers without human help. CSAT and satisfaction scores reveal what users actually think.
Retention rates don't lie – people return to bots that work. And let's not forget conversion rates.
Bot's job is to drive action, right? Sessions per user and goal completion rates complete the picture.
Numbers don't sugarcoat performance – they expose it.