Beautiful Soup simplifies web scraping in Python. First, install it with 'pip install beautifulsoup4' along with the requests library. Fetch HTML content using requests, then create a soup object with 'BeautifulSoup(html_content, 'html.parser')'. Extract data using 'find()' or 'find_all()' methods and get the text with 'get_text()'. Not magic for dynamic sites—you'll need Selenium for those. Follow website terms before going scrape-crazy. The real fun starts when you immerse yourself in the parsing details.
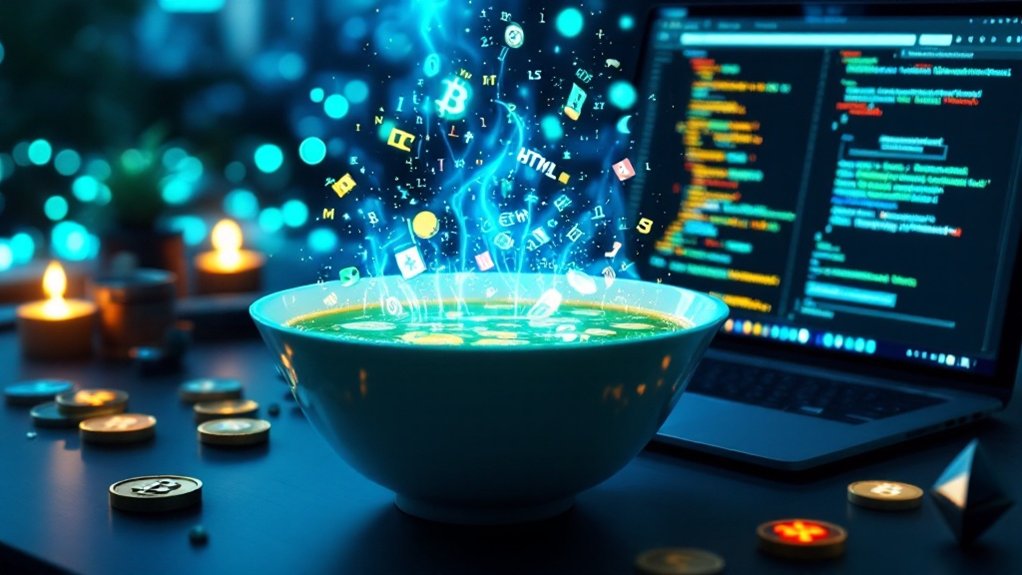
Every day, countless developers face the challenge of extracting data from websites. It's a common problem with a powerful solution: Beautiful Soup. This Python library transforms the headache of parsing HTML into something almost bearable. Almost.
Beautiful Soup specializes in creating parse trees from HTML documents, making data extraction straightforward. It's not magic—just good engineering. Developers use it primarily for web scraping static websites when APIs aren't available. Typical workflow? Fetch HTML, parse it, extract data, repeat. Simple as that.
No magic tricks, just parse trees that turn HTML chaos into orderly data extraction—what Beautiful Soup does best.
Getting started requires minimal setup. Just run 'pip install requests beautifulsoup4' and you're ready to go. The requests library handles the HTTP part, while Beautiful Soup does the heavy lifting with parsing. Some developers prefer virtual environments for installation. Smart choice. The data storage options include saving scraped information to CSV or Excel files for further analysis. Processing the scraped data through Z-score standardization ensures consistent scaling across different data sources.
The actual scraping process follows a predictable pattern. First, fetch the HTML content using requests. Then create a soup object with 'BeautifulSoup(html_content, 'html.parser')'. From there, it's a matter of using methods like 'find()' or 'find_all()' to locate specific tags. Want the text? Use 'get_text()'. Done. The three basic steps for web scraping include extracting HTML, analyzing the structure, and parsing with Beautiful Soup.
Beautiful Soup supports multiple parsers. The default 'html.parser' works fine, but 'lxml' is faster. Choice is yours.
Web scraping isn't without challenges. Dynamic JavaScript content? Beautiful Soup can't handle that alone—you'll need Selenium. Websites with anti-scraping measures? Good luck. Some sites really don't want you taking their data. Imagine that.
Best practices exist for a reason. Always check a website's terms of service. Use proper headers. Handle errors gracefully. Store data efficiently. Basic stuff, really.
The library excels at traversing HTML's DOM tree, letting developers extract precisely what they need. No more, no less. For those dealing with complex scraping tasks, Beautiful Soup remains an essential tool in the Python developer's arsenal. Not perfect, but damn useful. Always review a website's acceptable use policy before scraping to ensure you're following ethical scraping practices.
Frequently Asked Questions
How Do I Handle Javascript-Rendered Content With Beautiful Soup?
Beautiful Soup alone can't handle JavaScript-rendered content.
It's just a parser, not a browser. You need extra tools for that. Selenium is the go-to solution—it automates a real browser, executes the JavaScript, then hands the rendered HTML to Beautiful Soup for parsing.
Some developers use Playwright instead.
Another approach? Check if the site has an API. Or try inspecting network requests with developer tools.
JavaScript content isn't simple, but these workarounds get the job done.
Can Beautiful Soup Parse XML Files as Well as HTML?
Yes, Beautiful Soup handles XML files just fine. It's not just an HTML tool. Users need to specify "xml" as the parser feature when creating a BeautifulSoup object.
For best results, the lxml parser is recommended – it's faster and more efficient with XML structures. The parsing process works similarly to HTML, using familiar methods like find_all() to extract data.
XML has its own structure though, starting with that XML prolog thing.
How to Respect Robots.Txt When Scraping With Beautiful Soup?
Beautiful Soup doesn't handle robots.txt directly.
It's just a parser, not a crawler. Developers need to implement robots.txt compliance separately. The robotparser library in Python's urllib makes this easy.
First, check if URLs are allowed before scraping them. Simple as that.
'''python
from urllib.robotparser import RobotFileParser
rp = RobotFileParser()
rp.set_url("https://example.com/robots.txt")
rp.read()
if rp.can_fetch("MyBot", "https://example.com/page"):
proceed with Beautiful Soup scraping
'''
What's the Difference Between Find() and Select() Methods?
The difference? Pretty clear-cut. 'find()' grabs the first matching element and returns None if nothing's found. Simple, direct.
'select()' uses CSS selectors—more flexible—and returns a list of all matches, even if empty.
'find()' syntax: 'soup.find('div', {'class': 'header'})'
'select()' syntax: 'soup.select('.header')'
Performance-wise, 'find()' stops after one match. Faster.
'select()' checks everything. Better for complex queries. The choice depends on what you're after. Simple, right?
How to Handle Website Authentication When Scraping?
Beautiful Soup doesn't handle authentication. That's the cold truth. For protected content, developers need other tools.
Requests can manage basic auth and cookies. MechanicalSoup extends Beautiful Soup with form submission capabilities. For complex logins with JavaScript? Selenium's your answer. No getting around it.
Always inspect login forms first using browser developer tools. Session management is essential – cookies store those precious authentication tokens.
And please, respect website terms of service. Nobody likes a scraper who breaks rules.