Python offers three main ways to filter lists: list comprehensions, the filter() function, and traditional loops. List comprehensions are concise one-liners like [x for x in my_list if condition], while filter() pairs nicely with lambda functions for cleaner code. Traditional loops work too, just less elegant. Each method has its perks—comprehensions for readability, filter() for memory efficiency, loops for complex operations. The right choice depends on your specific needs and coding style. The journey continues with practical examples.
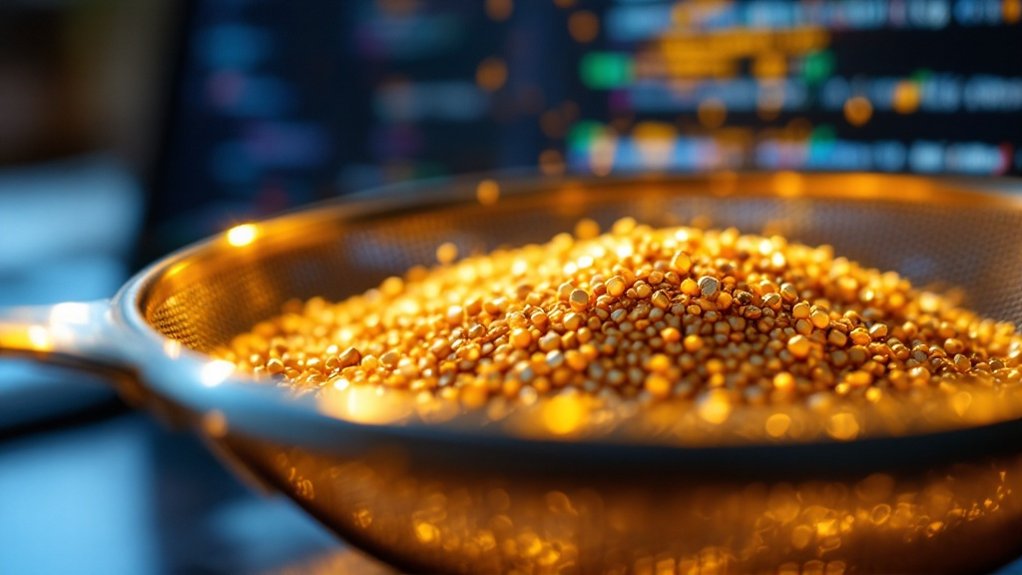
Filtering lists stands as a fundamental skill in Python programming. Anyone who's ever worked with data knows this truth: raw information is messy. Lists come packed with elements you don't need, and sometimes you just want the good stuff. Python, thankfully, offers several methods to separate the wheat from the chaff.
Raw data is messy. Python's list filtering capabilities cut through the noise, helping programmers extract only what matters.
Let's talk basics. Lists in Python are ordered collections that can hold different data types. They're flexible, mutable, and perfect for storing information that needs filtering. But why filter? Simple. Data processing demands precision. Nobody wants to wade through irrelevant information. Filtering cuts through the noise. Converting filtered lists to JSON strings enables seamless data exchange across different platforms. Much like data preparation in machine learning, filtering ensures your data is clean and properly formatted for further processing.
Python provides three main approaches to list filtering. First up: list comprehensions. These elegant one-liners follow a straightforward syntax: [expression for item in iterable if condition]. They're concise, efficient, and frankly, a bit sexy from a coding perspective. Want all even numbers from a list? One line does it. No fuss, no muss.
The filter() function offers another route. It takes two arguments—a function and an iterable—and returns only elements that pass the function's test. It's memory-efficient since it returns an iterator, not a list. Remember to convert it to a list if you want immediate results, though. Rookie mistake otherwise.
For the traditionalists, there's always the good old loop method. For loops with conditional statements get the job done, albeit less elegantly. They're like the reliable old car in your garage—not flashy, but they work. Plus, they're easier for beginners to understand and allow for more complex operations per iteration. When comparing these methods, the performance differences between simple loops, list comprehensions, and filter functions are typically marginal for most applications. They also excel when you need to handle exceptions within the filtering process.
Lambda functions often enter the filtering conversation too. These inline functions pair nicely with filter(), creating tidy solutions for straightforward filtering tasks. They're compact but powerful.
Each approach has its merits. The best choice? Depends on your needs, readability preferences, and performance requirements. Python's flexibility in list filtering is, frankly, one of its most practical features.
Frequently Asked Questions
How Does Filtering Affect Performance With Large Lists?
Filtering large lists hits performance hard. Memory usage spikes when storing filtered results.
List comprehensions? Generally faster than filter() function. Both still O(n) complexity though.
For massive datasets, traditional methods choke. No way around it.
Generators can save your RAM by processing items one-by-one. Chunking helps too.
Want serious speed? Multiprocessing distributes the workload. Or try specialized libraries like Dask. They're built for this stuff.
Can I Filter Lists of Custom Objects or Nested Structures?
Yes, Python handles filtering of custom objects and nested structures just fine. You can filter custom objects using their attributes through lambda functions or dedicated filter functions.
For nested structures, dot notation works well (obj.attribute.nested_attribute). List comprehensions make this pretty elegant. Just watch out for missing attributes—they'll crash your program without proper error handling.
Memory efficiency's a real bonus here, especially with filter() since it returns an iterator instead of creating a whole new list upfront.
Is Filtering Lists Thread-Safe in Python?
Standard list filtering operations in Python are not thread-safe. Period. When multiple threads access and modify the same list simultaneously, race conditions occur. The result? Unpredictable behavior, data corruption, or even crashes. No built-in protection here.
For thread-safe filtering, developers need explicit synchronization mechanisms like locks from the threading module. Alternatively, they can use thread-safe data structures from the queue module.
Python's GIL helps with atomic operations, but doesn't guarantee thread safety for complex operations.
How Do List Comprehensions Compare to Filter() for Memory Usage?
List comprehensions load everything into memory at once. Not great for huge datasets.
Filter(), on the other hand, creates an iterator – it processes elements one by one. Way more memory-efficient.
For small lists? Doesn't matter much. Both work fine.
But when you're dealing with massive data? Filter) wins hands down.
Funny how developers obsess over syntax preferences when memory usage might actually matter.
Pick the right tool for the job.
Can I Preserve the Original Indices After Filtering a List?
Yes, original indices can absolutely be preserved when filtering lists. Developers commonly use enumerate) for this purpose. It creates index-value pairs during filtering.
Example: [(i, x) for i, x in enumerate(my_list) if some_condition(x)]. This returns tuples with both original positions and values.
Pandas makes this even easier – its filtering operations naturally maintain index relationships.
For hardcore programmers, you could also build a dictionary mapping indices to values before filtering. Simple stuff, really.