Python data cleaning transforms messy datasets into reliable analysis foundations. Start by loading data with Pandas, then tackle the dirty work: missing values get dropped or filled, duplicates eliminated, and outliers tamed. Convert data types for consistency, standardize dates, and normalize text. One-hot encode those pesky categorical variables. Don't forget validation—document every cleaning step. The difference between garbage results and brilliant insights? Clean data.
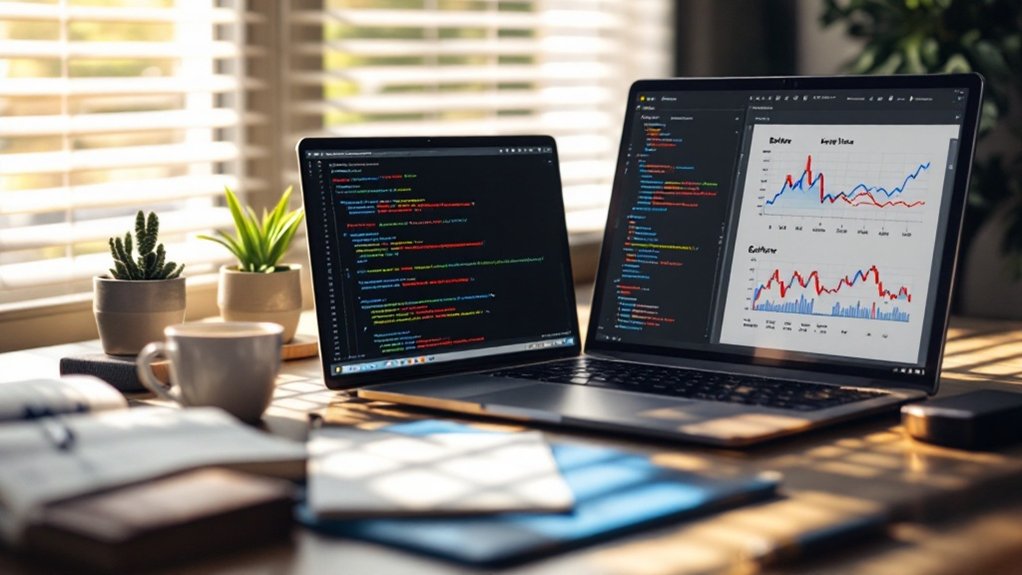
Garbage in, garbage out. Data scientists know this truth all too well. Raw datasets arrive messy, incomplete, and absolutely riddled with problems. Python offers powerful tools to whip these unruly datasets into shape. No clean data, no reliable analysis. Simple as that.
Pandas dominates the Python data cleaning landscape. Loading data is step one – CSV, Excel, databases, whatever. A quick df.head() shows what you're dealing with. Utilizing inner merge operations helps identify data inconsistencies when combining multiple sources. Check dtypes, run describe(), and count those missing values with isnull().sum(). Know your enemy before fighting it.
Missing data plagues every dataset. Deal with it. Drop rows entirely with dropna() if you can afford the loss. Otherwise, fillna) with means or medians. Some prefer interpolation or forward/backward filling. The sklearn library offers fancier imputation methods. Pick your poison. Regular scan monitoring of your dataset helps detect anomalies similar to how device scans identify suspicious patterns.
Missing values are inevitable. Deal with them strategically or watch your analysis crumble before your eyes.
Duplicates waste space and skew results. Find them with duplicated() and eliminate them with drop_duplicates). Sometimes keeping the first or last occurrence makes sense. Don't forget to reset that index afterward.
Outliers. They're the weirdos of your dataset. Spot them using IQR or z-scores. Box plots make them obvious. Remove them, cap them, or transform the data. Or just use robust methods that don't care about outliers. Your call.
Inconsistent formats will drive you insane. Convert data types with astype(). Standardize dates with to_datetime(). Text data needs normalization – lowercase everything, strip whitespace, kill those special characters. Categorical variables? One-hot encode them with get_dummies().
Text data needs special attention. Strip those spaces. Replace weird characters. Fix capitalization issues. Spell-checking helps too. NLP tasks require tokenization and lemmatization. It's worth the effort.
Always validate your cleaning work. Check data integrity. Verify relationships between variables. Document every cleaning step you take – future you will thank present you. Trust me.
Done right, data cleaning transforms garbage into gold. Skip it, and your analysis is worthless. That's just how it works. Creating reproducible workflows helps ensure consistent results across projects and makes your cleaning process scalable for team environments. When handling age or salary columns, watch for statistical outliers that can significantly affect your means and distributions.
Frequently Asked Questions
How to Handle Missing Categorical Data Differently Than Numerical Values?
Missing categorical data requires special treatment. While numeric values can use means or medians, categories don't average.
Options include creating an "Unknown" category, using mode imputation, or applying KNN methods to predict categories from similar records. Decision trees work well too.
Multiple imputation generates several plausible values, accounting for uncertainty. Always validate your approach.
Impact on downstream analysis matters more than the method itself. No one-size-fits-all solution exists.
Can Automated Data Cleaning Pipelines Replace Manual Inspection Entirely?
Automated pipelines? Not a total replacement for human eyes. Never.
They're efficient for routine cleaning and handling big data volumes, sure. But they miss nuanced issues.
Can't match human judgment for complex cases. Might accidentally trash valid outliers too.
The reality? A hybrid approach works best. Let machines handle the grunt work. Humans tackle the edge cases.
Domain expertise still matters. Always will.
What Performance Considerations Exist When Cleaning Large Datasets?
Cleaning large datasets isn't for the faint-hearted. Memory optimization is essential—convert columns to proper data types, drop unnecessary ones early.
Processing in chunks prevents RAM explosions. Vectorized operations crush loops every time. Strategic handling of missing values saves headaches.
For truly massive data? SQL databases or distributed computing with Dask or PySpark.
And please, monitor memory usage religiously. Nobody enjoys system crashes mid-cleaning.
How to Detect and Handle Outliers in Multivariate Data?
Detecting outliers in multivariate data requires specialized techniques.
Visualization methods like scatter plots and heat maps reveal anomalies visually.
Statistical approaches—Mahalanobis distance, Isolation Forest, Local Outlier Factor—identify points that deviate from expected patterns.
Machine learning tools like autoencoders and one-class SVMs excel at complex outlier detection.
Once identified, outliers can be removed, capped, transformed, or handled with robust methods.
No single approach works for all datasets. Choose accordingly.
When Should I Use Data Imputation Versus Dropping Incomplete Records?
Data imputation shines when missing values are MAR and preserving sample size matters.
Drop records when data is MCAR or the proportion of missing values is minimal. Simple as that.
Computational resources matter too—imputation can be intensive. The pattern of missingness is essential!
For MNAR data, neither approach works perfectly. Always analyze your missingness patterns first.
Multiple imputation? Worth considering for uncertainty quantification.