Building a Python API requires selecting the right framework—Flask for simplicity, Django for complexity, or FastAPI for speed. Set up a Python environment, define endpoints using decorators, and implement HTTP methods. Don't forget security: JWT authentication, HTTPS, and proper input validation are non-negotiable. Error handling and testing guarantee everything works as intended. Proper documentation through Swagger or ReDoc completes the package. The world of API development awaits.
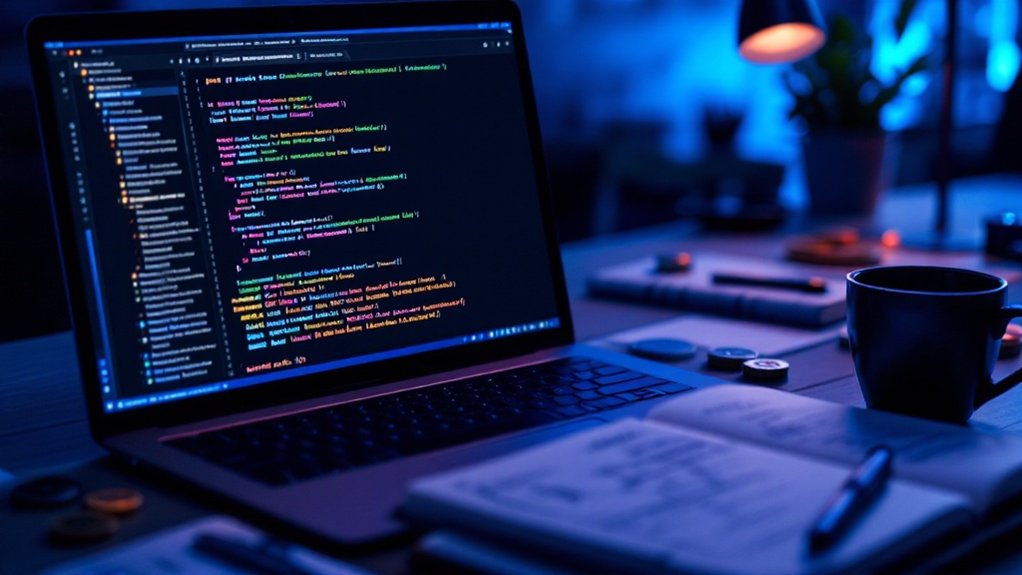
Building APIs in Python isn't rocket science. Developers have plenty of web frameworks to choose from, depending on their project's complexity. Flask works great for smaller projects—lightweight and flexible. Need something robust for complex applications? Django's your answer. FastAPI is the new kid on the block, built specifically for APIs and speed freaks. FastAPI was developed by Sebastián Ramírez in 2018 for Python and has gained popularity quickly. FastAPI automatically generates OpenAPI schemas from your Python code, making documentation effortless. There's also Pyramid for scalability and Tornado if you're after high performance. Pick one and move on.
Setting up your development environment requires minimal effort. Install Python 3.7 or newer, create a virtual environment (because nobody likes dependency hell), and install your chosen framework via pip. Similar to neural networks, proper setup is crucial for success. Much like implementing ChatterBot training, you'll need to set up essential libraries and configurations. Organize your project sensibly—separate routes from models. Connect to your database. Done.
Next comes designing API endpoints. Use decorators to specify routes. Implement the standard HTTP methods: GET, POST, PUT, DELETE. Nobody respects an API with sloppy URL structure, so make it RESTful. Handle parameters properly and use appropriate status codes. A 200 is not always the right answer.
Data models matter. Create classes that represent your data structures. ORMs save time when dealing with databases. Validate data input—garbage in, garbage out. Define relationships clearly. Serialize and deserialize data properly. It's not optional.
Security isn't an afterthought. Implement JWT for authentication. Use HTTPS—it's 2023, for crying out loud. Rate limiting prevents abuse. Validate input to avoid injection attacks. Set up proper CORS policies. Skip these steps at your peril.
Errors happen. Deal with them. Create custom exception classes. Implement global handlers. Return appropriate status codes with informative messages. Log everything for when things inevitably break.
Testing isn't just for perfectionists. Write unit and integration tests. Use Postman or cURL to test manually. Document your API with Swagger or ReDoc—your future self will thank you. Version your API because change is inevitable.
That's it. Your Python API is ready for the world.
Frequently Asked Questions
How to Secure My Python API Against Unauthorized Access?
Securing Python APIs against unauthorized access isn't rocket science.
Developers should implement JWT for authentication, validate all inputs, and use HTTPS encryption without exception.
Role-based access control keeps users in their lane. API keys work for simpler needs.
Rate limiting stops brute-force attempts cold. Regular security audits? Non-negotiable.
Everything gets logged. No exceptions. The internet's a dangerous neighborhood—lock your API doors tight.
Can I Test My API Without a Frontend Application?
Yes, testing APIs without frontends is totally common. Developers use specialized tools like Postman, cURL, or Insomnia to send requests directly to endpoints.
No fancy UI needed. Automated testing through pytest or other frameworks checks functionality without visual interfaces. It's actually better this way—faster development cycles, earlier bug detection, improved API design.
Many teams test APIs independently before frontend work even begins. Just send the requests and analyze responses. Simple.
How Do I Handle Version Control for My API?
API version control isn't rocket science.
Implement semantic versioning (Major.Minor.Patch) so users know what's changing. Add version numbers in URLs like /v1/endpoint, or use custom headers.
Multiple versions? No problem. Use feature flags and API gateways.
Plan ahead—design for extensibility and backward compatibility. Don't just pull the rug out from under users.
Deprecate old versions gradually, with clear timelines. Nobody likes surprise breaking changes.
What's the Best Way to Document a Python API?
Documenting Python APIs? Three words: docstrings are non-negotiable. They're your first line of defense.
Sphinx takes those docstrings and transforms them into something humans actually want to read. Best practice? Document as you code, not after.
Include examples—lots of them. Nobody understands abstract explanations. API documentation isn't a creative writing exercise; it's functional.
Clear request/response examples beat flowery descriptions every time. Version changes? Document those too.
How to Deploy a Python API to Production Environments?
Deploying Python APIs to production isn't rocket science. Containerize with Docker—package everything neatly and push to a registry.
Cloud platforms make life easier: AWS Elastic Beanstalk, Heroku, or Google App Engine handle the heavy lifting.
Server configuration matters: set up Nginx, configure WSGI servers like Gunicorn, and enable HTTPS.
Don't forget CI/CD pipelines. GitHub Actions or Jenkins automate deployment, saving precious time. No more manual uploading. Thank goodness.