Importing datasets in R isn't rocket science. Beginners can use read.csv) for basic CSV files or explore RStudio's Import Dataset button for a visual approach. For larger files, fread) from the data.table package prevents your computer from having a meltdown. Excel files? The readxl package has your back. Remember to set your working directory first with setwd). Different data sources require different tools. The journey continues with countless other data formats waiting to be accessed.
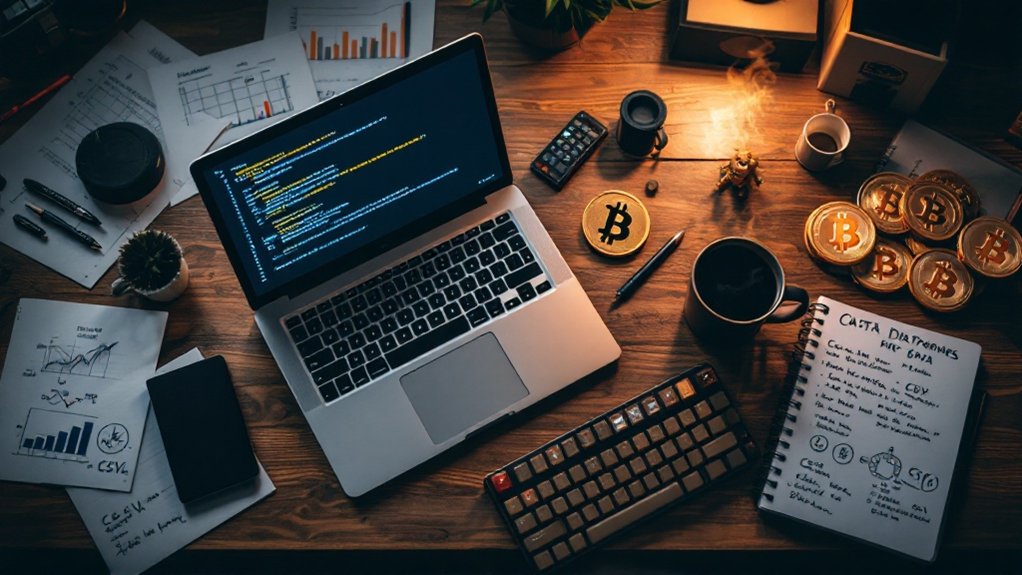
Importing data ranks as one of the most fundamental tasks in R programming. It's the gateway to analysis. Without data, you're just staring at a blank console, wondering why you downloaded R in the first place. Let's face it—you need to get your data in before the fun stuff happens.
R offers several ways to import CSV files, the bread and butter of data exchange. The classic function is read.csv), which works fine but isn't winning any speed competitions. Want something faster? Try read_csv) from the readr package. It's like the sports car version of data importing. Just point it to your file path and watch it go. Unless you mess up the path. Then you'll just get errors and frustration. Similar to how Beautiful Soup library parses HTML content, R's functions parse structured data files efficiently.
Text files aren't going anywhere either. Use read.table) or read.delim) depending on how your data is separated. Don't forget to specify your delimiter—commas, tabs, whatever weird character your data provider decided to use. Set header = TRUE if you want column names. Seems obvious, but people forget.
RStudio makes importing almost too easy. Click the "Import Dataset" button and follow the prompts. It even generates code for you. Copy, paste, done. The interface lets you preview data before committing—pretty handy when files are formatted by people who apparently hate data scientists. Use setwd() function to establish your working directory before importing files.
Got massive datasets? Regular functions might choke. Try fread) from data.table or stick with read_csv(). They're optimized for speed and memory. You can also use the ff package with read.table.ffdf() function to load data in chunks for better performance. Large files don't have to crash your system anymore.
Working with databases requires special packages like RMySQL or RPostgreSQL. You'll write SQL queries to grab just what you need. Security matters here—don't be the one who exposes database credentials in shared code.
Excel files? Easy. The readxl package handles them without complaint. Or just export to CSV first and avoid the whole mess. LibreOffice Calc works too, often better than Excel for data prep. Because sometimes simplicity wins.
Remember that when working with variables in data frames, you can use the attach() function to simplify access to variables by name without needing to reference the data frame each time.
Frequently Asked Questions
How Can I Troubleshoot Common Import Errors in R?
Troubleshooting import errors in R? Not rocket science. First, check file formats and paths. Slashes matter. Missing values should be marked "NA." Column names? Keep 'em simple, no weird characters. Data types need consistency.
Working directory issues trip up everyone. When functions throw errors, read the message. It's telling you something. Package problems? Install what you need first, then import.
R starts counting at 1, not 0. Remember that.
Can I Import Data Directly From Online Sources?
Absolutely. R makes online data importing surprisingly easy. Just use the URL directly in functions like read.csv() or fread). No downloading necessary.
RStudio even has a built-in interface for this – just click through "Import Dataset" from the Tools menu. The data.table package's fread() function works particularly well for large datasets.
Different file formats? No problem. R handles CSV, TXT, and other common types without breaking a sweat.
What's the Difference Between Read.Csv and Read_Csv Functions?
The difference is stark. read.csv) is base R, slower, and returns traditional data frames.
read_csv() comes from readr package, runs faster, and creates tibbles instead. Performance? No contest. read_csv) smokes the competition with large datasets.
It also handles strings better—no automatic conversion to factors. Plus, it gives clearer error messages and column specs.
Base function works fine though. No packages needed.
How Do I Handle Datasets With Missing Values?
Missing values in datasets? Not exactly a walk in the park. R offers multiple approaches to tackle them.
Use is.na() to identify these pesky gaps, then decide: remove them with na.omit) or complete.cases(), or fill them in.
Imputation methods include mean/median replacement, KNN, or multiple imputation via the mice package.
During import, the na.strings argument helps R recognize what counts as "missing."
Choose wisely—each method has consequences.
How Can I Import Excel Files With Multiple Sheets?
Importing Excel files with multiple sheets is simple in R. The readxl package makes it painless.
First, use excel_sheets) to see all sheet names. Then, either import sheets one by one with read_excel(), specifying the sheet parameter.
Or, use lapply) for all sheets at once, creating a neat list of data frames. Each approach works. Depends what you need.
No rocket science here.