Converting Jupyter notebooks to Python scripts is essential for production environments. Researchers can't run notebooks in real-world applications—too messy. Multiple methods exist: export via Jupyter's interface, use nbconvert command-line tools, or click convert in VS Code. Before conversion, clean up unnecessary debugging code and restructure into proper functions. Add error handling and implement conditional execution with "if __name__ == '__main__'". The difference between experimental notebooks and production-ready scripts might shock you.
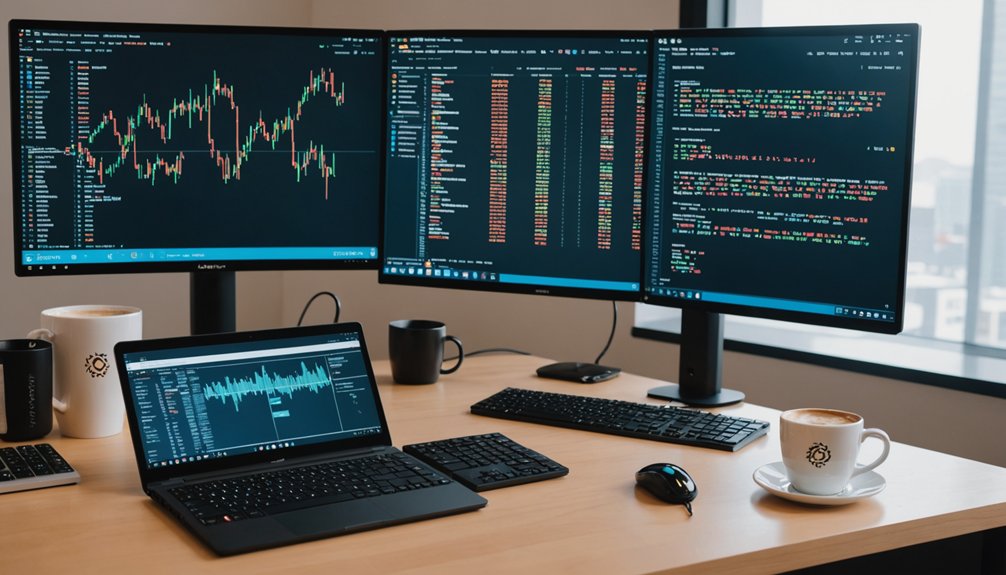
While Jupyter notebooks offer a fantastic interactive environment for data exploration and visualization, they aren't always practical for production environments. Let's be real—at some point, you need actual scripts. That's just how it works in the real world. Running notebooks in production? Nightmare fuel. The Markdown cells feature makes notebooks great for documentation, but this isn't necessary in production scripts.
Converting notebooks to Python scripts isn't rocket science. There are multiple ways to do it. The Jupyter interface itself lets you export through the "File" menu—just select "Save and Export Notebook as…" followed by "Executable Script." Simple. The nbconvert package does the same thing via command line. Even VS Code has a convert button. Pick your poison. Consider using ChatterBot libraries for any conversational AI components in your scripts.
But conversion alone isn't enough. Notebooks are usually messy. Too much print debugging. Pointless outputs everywhere. Before converting, clean that mess up! Remove unnecessary print statements. Keep only essential code. Your future self will thank you. Trust me.
The real magic happens after conversion. Restructure that spaghetti code into proper functions. Use conditional execution with "if __name__ == '__main__'". It's basic Python etiquette, people. This makes your code reusable and easier to maintain. No more scrolling through endless lines trying to find that one change.
For production environments, especially clusters, you'll need proper input/output handling. Argparse is your friend here. File paths? Always a headache. Use environment variables or command-line arguments to manage them dynamically. And for pete's sake, add some error handling! You can also implement multiple input files handling with nargs='+' for batch processing needs. Creating functions like 'split_data' and 'train_model' will significantly improve code maintainability and testing capabilities.
Running on clusters requires extra steps. Containerize your environment with Docker or Singularity. Create scheduler scripts with Slurm or similar tools. Define your resource needs explicitly—CPU, memory, the works.
Bottom line: notebooks are great for exploration; scripts are essential for production. The shift doesn't have to be painful. Clean, convert, refactor, enhance. That's the formula. No shortcuts.
Frequently Asked Questions
Can I Batch Convert Multiple Notebooks Simultaneously?
Yes, batch conversion of multiple notebooks is definitely possible.
Users can employ wildcards in their command. Simple as that. Just navigate to the notebook directory and run "jupyter nbconvert –to script *.ipynb" to convert all notebooks at once.
Works on Windows and Unix systems alike. The command processes everything matching the pattern. Efficient. No need to convert files one by one. Time saved.
How Do I Handle Interactive Visualizations in Converted Scripts?
Interactive visualizations often break during notebook-to-script conversion. Plotly and Bokeh work best since they support both environments.
Scripts need additional code to handle widgets and user inputs. For maximum flexibility, developers can save visualizations as HTML files or use Streamlit to convert their scripts into web applications.
Not all interactivity survives the shift, though. Sometimes you'll need custom JavaScript or specialized libraries to maintain functionality.
Will Magic Commands Work in the Converted Python Script?
Magic commands won't work in converted Python scripts. Period.
These IPython-specific shortcuts like %matplotlib inline are useless outside the Jupyter environment. They'll either cause errors or be ignored completely.
Developers need to replace them with standard Python equivalents or just remove them. Some conversion tools attempt to handle this automatically, but they're not perfect.
Manual cleanup is usually necessary. No magic in regular Python—just cold, hard code.
Can I Automate Notebook Conversion in a Ci/Cd Pipeline?
Yes, notebook conversion can be fully automated in CI/CD pipelines.
Tools like nbconvert integrate seamlessly with Jenkins, GitLab CI, or GitHub Actions. Simply add a conversion step in your pipeline configuration.
Scripts can handle the dirty work—installing dependencies, running conversions, and testing outputs. It's not rocket science.
Most teams use bash scripts or YAML configs to execute the conversions automatically whenever changes are pushed.
Efficiency at its finest.
How Do I Preserve Markdown Documentation in Converted Scripts?
Preserving markdown documentation when converting notebooks to scripts isn't rocket science. Jupytext does it automatically—markdown becomes Python comments right out of the box.
For nbconvert users, a custom template is needed. The Jupyter UI handles this too, though results vary.
Manual extraction? Time-consuming but effective.
No matter the method, documentation integrity remains critical. Without it, future developers are left in the dark. They'll hate that.